yolov8模型部署到web端
时间: 2023-12-16 19:05:44 浏览: 775
yolov8模型可以通过web部署来实现在线目标检测和分割。以下是yolov8模型部署到web端的步骤:
1. 准备模型文件和权重文件,可以通过yolov8系列的训练教程得到。
2. 安装必要的Python库,例如Flask、OpenCV等。
3. 编写Flask应用程序,实现模型的加载和推理功能。可以使用OpenCV库读取图像,然后调用模型进行目标检测和分割,最后将结果返回给前端。
4. 编写前端界面,可以使用HTML、CSS和JavaScript等技术实现。可以使用Ajax技术将图像上传到后端进行处理,并将处理结果显示在前端界面上。
下面是一个简单的yolov8模型部署到web端的示例代码:
```python
# 导入必要的库
from flask import Flask, request, jsonify
import cv2
import numpy as np
# 加载模型和权重文件
net = cv2.dnn.readNetFromDarknet('yolov8.cfg', 'yolov8.weights')
# 定义Flask应用程序
app = Flask(__name__)
# 定义处理图像的函数
def process_image(image):
# 调整图像大小
resized_image = cv2.resize(image, (416, 416))
# 将图像转换为blob格式
blob = cv2.dnn.blobFromImage(resized_image, 1/255.0, (416, 416), swapRB=True, crop=False)
# 设置网络的输入
net.setInput(blob)
# 进行前向推理
outputs = net.forward()
# 解析输出结果
boxes, confidences, class_ids = [], [], []
for output in outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x, center_y, width, height = list(map(int, detection[0:4] * [image.shape[1], image.shape[0], image.shape[1], image.shape[0]]))
x, y = center_x - width // 2, center_y - height // 2
boxes.append([x, y, width, height])
confidences.append(float(confidence))
class_ids.append(class_id)
# 进行非极大值抑制
indices = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
# 绘制检测结果
for i in indices:
i = i[0]
x, y, w, h = boxes[i]
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.putText(image, str(class_ids[i]), (x, y - 5), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 返回处理后的图像
return image
# 定义Flask路由
@app.route('/detect', methods=['POST'])
def detect():
# 读取上传的图像
image = cv2.imdecode(np.fromstring(request.files['image'].read(), np.uint8), cv2.IMREAD_COLOR)
# 处理图像
result = process_image(image)
# 将处理结果转换为JPEG格式
_, jpeg = cv2.imencode('.jpg', result)
# 返回处理结果
return jsonify({'image': jpeg.tobytes()})
# 启动Flask应用程序
if __name__ == '__main__':
app.run()
```
阅读全文
相关推荐
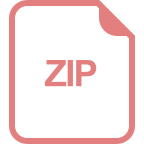
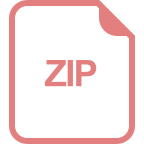
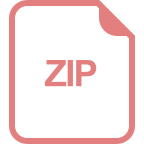
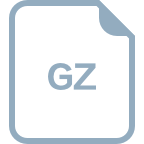
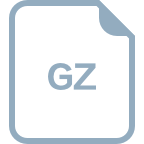
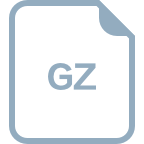
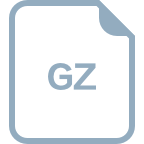
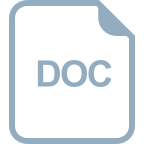
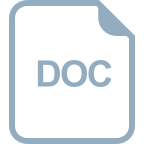
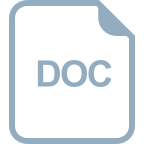
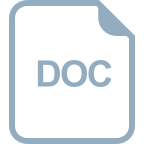
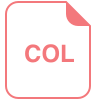
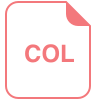




