编程一个可以输入包含多个视频的文件夹,然后依次把每个视频逐帧提取文字,并将每帧的文字作为一个句子。接着,比较相邻帧的句子内容,如果内容完全相同,则只保留其中一个。并以表格形式输出,以及翻译一份英文办的word的python程序
时间: 2024-09-24 11:10:17 浏览: 43
为了实现这个功能,你需要用到Python的一些库,如`moviepy`, `opencv-python`, `tesseract-ocr`, 和 `pandas` 等。这里是一个简单的步骤概述:
1. **安装所需库**:
```
pip install moviepy opencv-python pytesseract pandas
```
2. **读取视频文件并逐帧提取文字**:
使用`moviepy.editor`库来处理视频文件,例如:
```python
from moviepy.editor import VideoFileClip
import cv2
def extract_text_per_frame(video_path):
clip = VideoFileClip(video_path)
text_frames = []
for frame in clip.iter_frames():
gray_image = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 使用Tesseract OCR识别文字
ocr_text = pytesseract.image_to_string(gray_image)
text_frames.append(ocr_text)
return text_frames
```
3. **比较相邻帧并去重**:
```python
def remove_duplicates(text_frames):
unique_sentences = [text_frames[0]]
for i in range(1, len(text_frames)):
if text_frames[i] != text_frames[i - 1]:
unique_sentences.append(text_frames[i])
return unique_sentences
```
4. **创建表格并输出结果**:
```python
import pandas as pd
def create_dataframe(unique_sentences):
df = pd.DataFrame({'Frame': list(range(len(unique_sentences))), 'Sentence': unique_sentences})
return df
```
5. **翻译英文Word文档**:
这部分需要使用`python-docx`库来操作Word文件,然后结合Google Translate API或其他在线翻译服务(注意需自行注册获取API密钥):
```python
from docx import Document
from googletrans import Translator
def translate_word_document(word_doc_path):
translator = Translator()
translated_text = []
with open(word_doc_path, 'r', encoding='utf-8') as doc_file:
for paragraph in doc_file.read().split('\n'):
translation = translator.translate(paragraph).text
translated_text.append(translation)
return translated_text
```
6. **整合所有功能并运行**:
```python
video_folder = "path/to/videos" # 输入视频文件夹路径
word_doc_path = "path/to/document.docx" # 输入Word文档路径
video_files = os.listdir(video_folder) # 获取视频文件列表
for file_name in video_files:
# 提取视频文字,翻译Word文档,去除重复
video_text = extract_text_per_frame(os.path.join(video_folder, file_name))
translated_text = translate_word_document(word_doc_path)
unique_video_text = remove_duplicates(video_text)
# 输出表格
result_df = create_dataframe(unique_video_text)
result_df.to_csv(f"{file_name[:-4]}_results.csv", index=False)
print("任务完成!")
```
记得替换上述代码中的路径为你实际的文件位置,同时要注意Tesseract和Google Translate可能需要适当的设置才能正常工作。
阅读全文
相关推荐
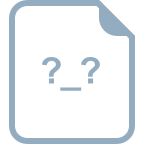
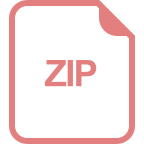
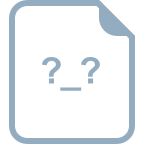
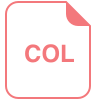
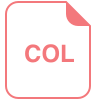
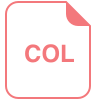
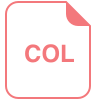
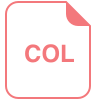


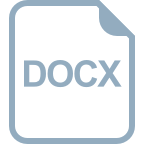
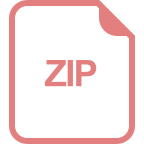
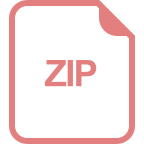
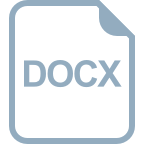
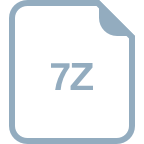
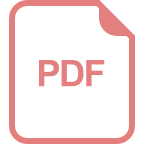