R语言中如何读取CSV文件并进行初步的数据清洗?
时间: 2024-09-27 22:08:18 浏览: 53
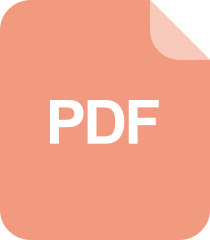
R语言数据分析案例分析一个关于房价的数据集.pdf
在R语言中,读取CSV文件通常使用`read.csv()`函数,这是一个非常基础的操作。例如,如果你想读取名为"data.csv"的文件,你可以这样做:
```R
data <- read.csv("data.csv")
```
对于初步的数据清洗,R提供了许多内置函数和包来进行处理。下面是一些常见的步骤:
1. 检查数据结构和完整性:
```R
str(data) # 查看数据的结构
summary(data) # 简单描述性统计
```
2. 缺失值处理:
```R
na.omit(data) # 删除包含缺失值的行
data[is.na(data)] <- NA # 将缺失值替换为NA
```
3. 异常值检测和处理:
```R
boxplot(data) # 绘制箱线图检查异常值
outliers <- boxplot.stats(data)$out # 找到可能的离群点
data[outliers] <- NA # 或者用其他方法处理
```
4. 数据类型转换:
```R
data$column_name <- as.numeric(data$column_name) # 如果需要将列转为数值型
```
5. 列名标准化或统一:
```R
names(data) <- tolower(names(data)) # 转换列名为小写
```
记得在实际操作前备份原始数据,并根据具体情况调整清洗策略。
阅读全文
相关推荐
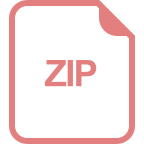
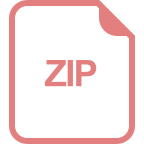
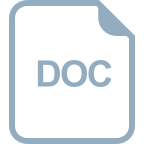
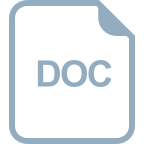
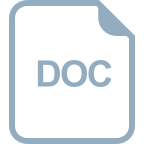
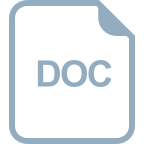
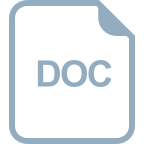
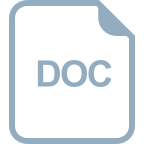
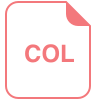
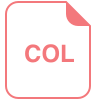
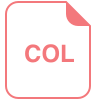
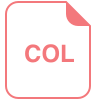
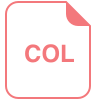
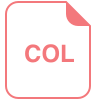
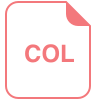
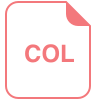
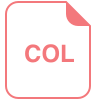
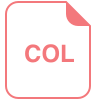