websocketpp 服务器断开重连
时间: 2024-02-29 11:50:03 浏览: 37
WebSocket++是一个C++库,用于实现WebSocket协议的客户端和服务器端。它提供了一种简单而灵活的方式来创建WebSocket连接,并支持服务器断开重连的功能。
要实现WebSocket++服务器断开重连的功能,可以按照以下步骤进行操作:
1. 创建WebSocket连接:使用WebSocket++库创建一个WebSocket连接对象,并指定服务器的地址和端口号。
2. 设置回调函数:为WebSocket连接对象设置回调函数,以便在连接状态发生变化时进行处理。可以设置连接成功、连接关闭、连接错误等不同状态的回调函数。
3. 连接服务器:使用WebSocket连接对象的connect()函数连接到服务器。
4. 处理连接状态:在回调函数中处理连接状态的变化。当连接关闭时,可以在回调函数中进行重连操作。
5. 断开重连:在连接关闭的回调函数中,使用定时器或其他方式进行断开重连操作。可以设置一个重连的时间间隔,定时尝试重新连接服务器。
下面是一个示例代码,演示了如何使用WebSocket++库实现服务器断开重连的功能:
```cpp
#include <websocketpp/client.hpp>
typedef websocketpp::client<websocketpp::config::asio_client> client;
class WebSocketClient {
public:
WebSocketClient() {
// 设置连接成功的回调函数
m_client.set_open_handler([this](websocketpp::connection_hdl hdl) {
m_connection = hdl;
std::cout << "Connected to server" << std::endl;
});
// 设置连接关闭的回调函数
m_client.set_close_handler([this](websocketpp::connection_hdl hdl) {
std::cout << "Disconnected from server" << std::endl;
// 在连接关闭时进行断开重连操作
reconnect();
});
// 设置连接错误的回调函数
m_client.set_fail_handler([this](websocketpp::connection_hdl hdl) {
std::cout << "Connection failed" << std::endl;
// 在连接错误时进行断开重连操作
reconnect();
});
}
void connect(const std::string& serverAddress, int port) {
std::string uri = "ws://" + serverAddress + ":" + std::to_string(port);
m_client.init_asio();
websocketpp::lib::error_code ec;
client::connection_ptr con = m_client.get_connection(uri, ec);
m_client.connect(con);
m_client.run();
}
void reconnect() {
// 在这里进行断开重连操作,可以使用定时器等方式
std::cout << "Reconnecting to server..." << std::endl;
// 重新连接服务器
connect(m_serverAddress, m_port);
}
private:
client m_client;
websocketpp::connection_hdl m_connection;
std::string m_serverAddress;
int m_port;
};
int main() {
WebSocketClient client;
client.connect("localhost", 8080);
return 0;
}
```
在上面的示例代码中,WebSocketClient类封装了WebSocket++库的相关功能。在构造函数中设置了连接成功、连接关闭和连接错误的回调函数。在连接关闭和连接错误的回调函数中调用了reconnect()函数进行断开重连操作。
你可以根据自己的需求修改和扩展上面的示例代码,以满足你的具体需求。
相关推荐
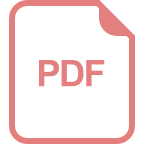
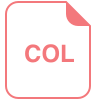
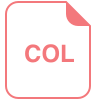
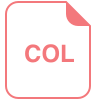











