使用pythonBoston对于Boston数据集,编程实现数据的二维显示:折线图、散点图、饼图显示等。数据集
时间: 2024-10-12 15:07:58 浏览: 26
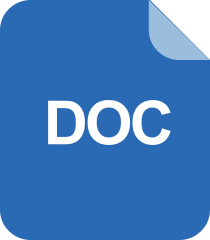
MATLAB课程设计

在Python中,我们可以使用`pandas`库处理`boston`数据集,然后利用`matplotlib`和`seaborn`库来进行二维数据可视化。首先,你需要导入必要的库并加载数据:
```python
import pandas as pd
import seaborn as sns
import matplotlib.pyplot as plt
# 加载波士顿房价数据集
from sklearn.datasets import load_boston
boston = load_boston()
df_boston = pd.DataFrame(boston.data, columns=boston.feature_names)
df_boston['PRICE'] = boston.target # 添加目标变量(房价)
# 对于二维数据展示,我们假设你想看某一特征与价格的关系
feature_of_interest = 'RM' # 房间的数量
```
接下来,你可以按照以下步骤创建不同的图形:
1. **折线图**(Line plot):
```python
plt.figure(figsize=(8,6))
sns.lineplot(x=feature_of_interest, y='PRICE', data=df_boston)
plt.title(f'Boston Housing Dataset: Price vs. {feature_of_interest}')
plt.xlabel(feature_of_interest)
plt.ylabel('Price')
plt.show()
```
2. **散点图**(Scatter plot):
```python
plt.figure(figsize=(8,6))
sns.scatterplot(x=feature_of_interest, y='PRICE', data=df_boston)
plt.title(f'Scatter Plot: Price vs. {feature_of_interest}')
plt.xlabel(feature_of_interest)
plt.ylabel('Price')
plt.show()
```
3. **饼图**(Pie chart),通常用于分类数据,如果你想要显示某个特征的分布对价格的影响可能不太合适,因为它们不是相互独立的关系。但如果有的话,例如查看不同房型(如‘RM’有不同取值段)的价格占比:
```python
feature_groups = df_boston[feature_of_interest].value_counts().sort_index()
fig, ax = plt.subplots(figsize=(6,6))
ax.pie(feature_groups, labels=feature_groups.index, autopct='%1.1f%%')
plt.title(f'Distribution of {feature_of_interest} in Boston Housing Data')
plt.show()
```
请注意,这些示例假设`feature_of_interest`是一个数值型特征。如果它是分类特征,那么饼图将是合适的,而线图和散点图则需要调整。
阅读全文
相关推荐
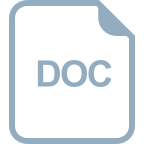
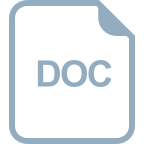
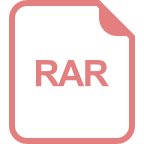
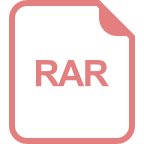
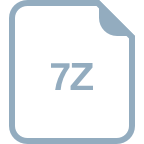
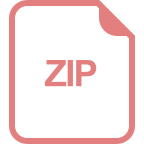
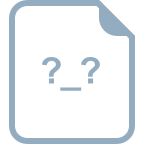
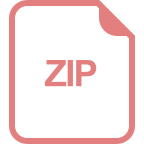
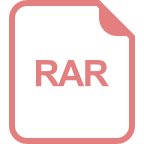
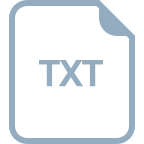
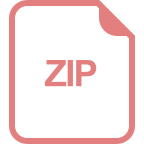
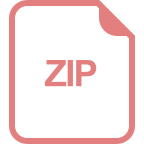
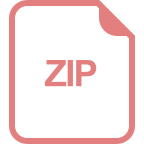
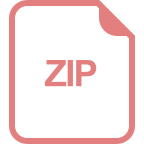
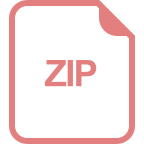
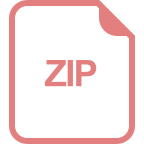
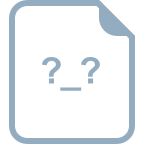
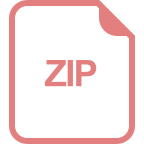