实现一个命令行文本计数统计程序。能正确统计导入的纯英文txt文本中的字符数,单词数,句子数。
时间: 2024-10-27 08:05:20 浏览: 96
实现一个命令行文本计数统计程序通常涉及以下几个步骤:
1. **读取文件**:首先需要从用户输入或指定的路径读取.txt文本文件。可以使用操作系统提供的API(如Python的`open()`函数)来完成。
```python
def read_text(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
```
2. **处理文本**:
- **字符计数**:计算所有非空格字符的数量,包括字母、数字、标点符号等。
- **单词计数**:分割文本成单词列表,去除空格和特殊字符后统计单词数量。可以使用正则表达式或内置的字符串方法(如Python的`split()`)。
- **句子计数**:一般认为句子是以句号`.`、问号`?`或感叹号`!`结尾的非空白段落。遍历文本并检查每个分隔后的部分。
```python
import re
def count_elements(text):
char_count = len(text)
word_list = re.findall(r'\b\w+\b', text.lower())
word_count = len(word_list)
# 句子计数,这里简化了判断,仅以句点为准
sentence_list = text.split('. ')
sentences = [sent for sent in sentence_list if sent]
sentence_count = len(sentences)
return char_count, word_count, sentence_count
```
3. **显示结果**:将计算得到的字符数、单词数和句子数打印到控制台。
```python
def print_counts(char_count, word_count, sentence_count):
print(f"字符数: {char_count}")
print(f"单词数: {word_count}")
print(f"句子数: {sentence_count}")
```
4. **主程序**:整合上述功能,接收用户输入的文件路径,并调用相应的函数。
```python
if __name__ == "__main__":
file_path = input("请输入文本文件路径:")
text = read_text(file_path)
char_count, word_count, sentence_count = count_elements(text)
print_counts(char_count, word_count, sentence_count)
```
阅读全文
相关推荐
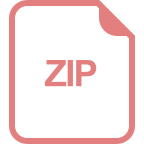
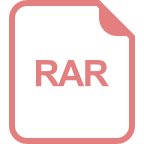

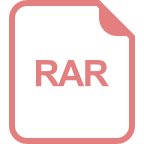
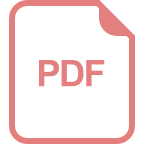
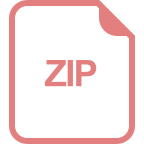
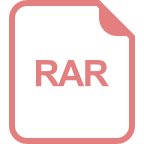
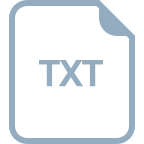
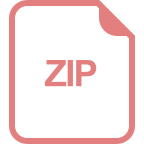
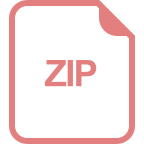
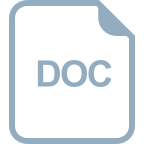
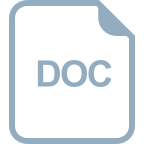
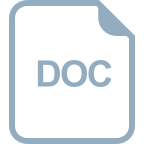
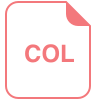




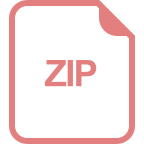