java连接mysql数据库失败
时间: 2023-12-23 08:26:54 浏览: 212
以下是Java连接MySQL数据库失败的可能原因及解决方法:
1. 数据库名字错误导致的连接失败:
当控制台报错信息中有:Unknown database ‘*****’,这句话的意思是,数据库名字错误,也就是说你代码里设置的数据库名字和在MySQL中真实的数据库名字不一样。解决方法是检查代码中的数据库名字是否正确。
2. 数据库未启动导致的连接失败:
当控制台报错信息中有:Communications link failure Last packet sent to the server was 0 ms ago. com.mysql.jdbc.exceptions.jdbc4.CommunicationsException: Communications link failure,这句话的意思是MySQL没有启动。解决方法是检查MySQL是否已经启动。
3. 密码错误导致的连接失败:
有时候,明明你在代码里设置了密码,但是运行还是提示密码错误,这可能是因为缓存导致的,你的改动并没有被识别。解决方法有两种:
- 重启IDE或者电脑,清除缓存。
- 在代码中添加以下语句,强制刷新缓存:
```java
DriverManager.getConnection(url, username, password);
```
相关问题
Java连接MySQL数据库
连接MySQL数据库需要使用JDBC(Java Database Connectivity)驱动程序。以下是一个简单的Java程序,演示如何连接MySQL数据库:
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class MySQLConnection {
public static void main(String[] args) {
Connection conn = null;
try {
// 加载MySQL驱动程序
Class.forName("com.mysql.jdbc.Driver");
// 数据库连接字符串
String url = "jdbc:mysql://localhost:3306/mydatabase";
// 数据库用户名
String user = "root";
// 数据库密码
String password = "mypassword";
// 获取数据库连接
conn = DriverManager.getConnection(url, user, password);
System.out.println("数据库连接成功!");
} catch (ClassNotFoundException e) {
System.out.println("找不到MySQL驱动程序!");
e.printStackTrace();
} catch (SQLException e) {
System.out.println("数据库连接失败!");
e.printStackTrace();
} finally {
// 关闭数据库连接
if (conn != null) {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
}
```
需要注意的是,此程序中的数据库连接字符串 `jdbc:mysql://localhost:3306/mydatabase` 中的 `mydatabase` 应替换为实际的数据库名称。同时,还需要将MySQL驱动程序的JAR文件添加到项目的classpath中。
java连接mysql数据库实现登录注册_java利用jdbc连接Mysql数据库——实现登录注册功能...
好的,我会尽量回答你的问题。关于Java连接MySQL数据库实现登录注册功能,以下是一个简单的实现步骤:
1.下载并安装MySQL数据库,安装完成后,创建一个名为“test”的数据库。
2.下载并安装JDBC驱动程序,将下载的JDBC驱动程序的jar包添加到项目的classpath中。
3.编写Java代码,实例化JDBC驱动程序,连接MySQL数据库,创建用户表,实现用户的注册和登录功能。
以下是一个简单的Java代码示例:
```
import java.sql.*;
public class JdbcTest {
public static void main(String[] args) {
// 数据库连接信息
String url = "jdbc:mysql://localhost:3306/test?useSSL=false&serverTimezone=UTC";
String username = "root";
String password = "123456";
// 注册JDBC驱动程序
try {
Class.forName("com.mysql.cj.jdbc.Driver");
} catch (ClassNotFoundException e) {
System.out.println("找不到JDBC驱动程序!");
e.printStackTrace();
return;
}
// 连接MySQL数据库
Connection conn = null;
try {
conn = DriverManager.getConnection(url, username, password);
} catch (SQLException e) {
System.out.println("连接MySQL数据库失败!");
e.printStackTrace();
return;
}
// 创建用户表
Statement stmt = null;
try {
stmt = conn.createStatement();
String sql = "create table if not exists user(id int primary key auto_increment, username varchar(50) not null, password varchar(50) not null)";
stmt.executeUpdate(sql);
} catch (SQLException e) {
System.out.println("创建用户表失败!");
e.printStackTrace();
return;
} finally {
if (stmt != null) {
try {
stmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// 用户注册
PreparedStatement pstmt = null;
try {
String sql = "insert into user(username, password) values (?, ?)";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, "user1");
pstmt.setString(2, "123456");
pstmt.executeUpdate();
} catch (SQLException e) {
System.out.println("用户注册失败!");
e.printStackTrace();
return;
} finally {
if (pstmt != null) {
try {
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// 用户登录
ResultSet rs = null;
try {
String sql = "select * from user where username=? and password=?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, "user1");
pstmt.setString(2, "123456");
rs = pstmt.executeQuery();
if (rs.next()) {
System.out.println("用户登录成功!");
} else {
System.out.println("用户名或密码错误!");
}
} catch (SQLException e) {
System.out.println("用户登录失败!");
e.printStackTrace();
} finally {
if (rs != null) {
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (pstmt != null) {
try {
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// 关闭数据库连接
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
在上面的代码中,我们先注册了JDBC驱动程序,然后连接了MySQL数据库,接着创建了一个名为“user”的用户表,实现了用户的注册和登录功能。你可以根据自己的需求修改代码中的数据库连接信息和SQL语句。
阅读全文
相关推荐
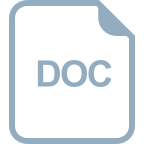
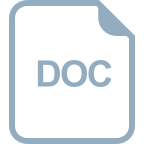
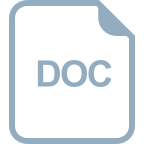
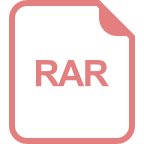
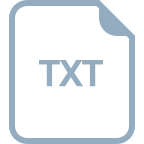
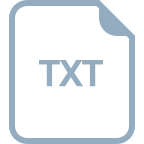
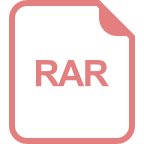
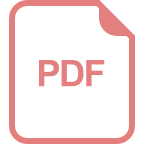
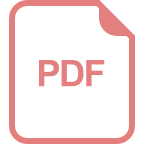
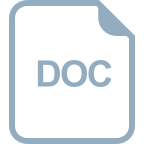
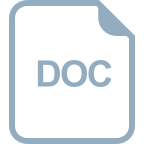




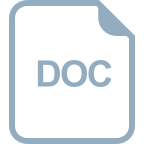