A*算法求解迷宫寻路问题实验pycharm运行结果
时间: 2024-10-07 13:08:26 浏览: 68
A*算法是一种启发式搜索算法,常用于解决像迷宫寻路这样的最短路径问题。在PyCharm中进行A*算法实验,首先你需要做的是:
1. **设置环境**:安装Python、PyCharm以及必要的科学计算库(如NumPy、Pandas等),还要安装图形化库(如pygame或matplotlib)用于显示迷宫和路径。
2. **编写代码**:创建一个`AStarSolver`类,其中包含计算起点到终点的最短路径函数。这个函数会利用开闭列表(open list和closed list)存储节点信息,同时结合启发式函数(如曼哈顿距离或欧几里得距离)评估每个节点的成本。
```python
class AStarSolver:
def __init__(self, heuristic_func):
self.heuristic_func = heuristic_func
self.open_list = []
self.closed_list = []
def find_path(self, start, end, maze):
# 初始化节点
start.g = 0
start.h = self.heuristic_func(start, end)
start.f = start.g + start.h
self.open_list.append(start)
while self.open_list:
current = self.get_lowest_f_cost_node()
if current == end:
return self.reconstruct_path(current)
self.close_node(current)
for neighbor in self.get_neighbors(maze, current):
tentative_g = current.g + 1
if neighbor not in self.closed_list or tentative_g < neighbor.g:
neighbor.g = tentative_g
neighbor.h = self.heuristic_func(neighbor, end)
neighbor.f = neighbor.g + neighbor.h
if neighbor not in self.open_list:
self.open_list.append(neighbor)
else:
self.open_list.remove(neighbor)
self.open_list.append(neighbor) # 保持排序
# ...其他辅助方法...
```
3. **测试和可视化**:在主程序中,实例化`AStarSolver`并传入迷宫矩阵,然后调用`find_path`获取路径。最后,你可以用pygame或matplotlib绘制出迷宫和找到的路径。
运行PyCharm后,你会看到初始状态的迷宫和逐渐生成的最优路径。需要注意的是,A*的结果依赖于所选的启发式函数的准确性和算法的具体实现。
阅读全文
相关推荐
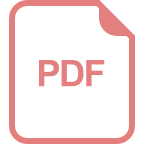
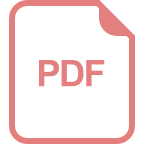
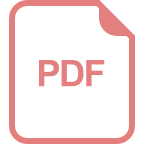
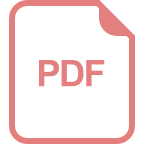
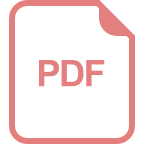
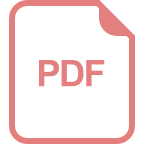
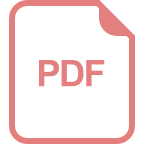
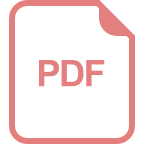
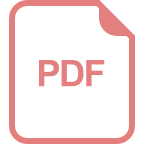
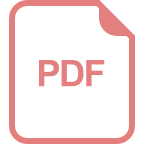
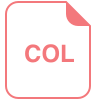