java 时间戳字符串比较
时间: 2024-12-27 07:20:38 浏览: 2
### 如何在 Java 中比较时间戳字符串
为了有效地比较两个时间戳字符串,在 Java 中通常需要先将这些字符串解析为 `Date` 或者 `Instant` 对象,然后再执行相应的比较操作。以下是具体方法:
#### 方法一:使用 SimpleDateFormat 解析并比较
如果时间戳是以特定日期格式存储的字符串,则可以利用 `SimpleDateFormat` 类来完成这一任务。
```java
import java.text.ParseException;
import java.text.SimpleDateFormat;
public class CompareTimestamps {
public static void main(String[] args) throws ParseException {
String timestampStr1 = "2023-09-15T14:48:00";
String timestampStr2 = "2023-09-16T14:48:00";
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss");
// 将字符串转换成 Date 对象以便于比较
java.util.Date date1 = sdf.parse(timestampStr1);
java.util.Date date2 = sdf.parse(timestampStr2);
int result = date1.compareTo(date2);
if (result < 0){
System.out.println("timestampStr1 is earlier than timestampStr2.");
} else if(result == 0){
System.out.println("Both timestamps are equal.");
} else{
System.out.println("timestampStr1 is later than timestampStr2.");
}
}
}
```
这种方法适用于处理固定格式的时间戳字符串[^1]。
#### 方法二:对于纯数字形式的时间戳(毫秒级)
当面对的是由 Unix 时间戳构成的字符串时——即表示自纪元以来经过了多少毫秒的一个整数值作为字符串的形式,可以直接将其转化为 `long` 类型再做对比。
```java
public class CompareUnixTimestampStrings {
public static void compare(long tsString1, long tsString2) {
Long t1 = Long.parseLong(tsString1+"");
Long t2 = Long.parseLong(tsString2+"");
int comparisonResult = t1.compareTo(t2);
if(comparisonResult<0){
System.out.println("tsString1 represents an earlier point in time compared to tsString2.");
}else if(comparisonResult==0){
System.out.println("The two provided timestamps represent the same moment in time.");
}else{
System.out.println("tsString1 represents a more recent point in time relative to tsString2.");
}
}
public static void main(String[] args) {
compare(1694772000L, 1694858400L); // Example usage with millisecond precision.
}
}
```
此方式适合用于那些仅包含毫秒级别精度的时间戳字符串之间的直接比较[^4]。
#### 方法三:采用 Instant 和 Duration 进行更精确的操作
针对更高版本 JDK 用户来说,推荐使用 `Instant` 结合 `Duration.between()` 来计算两者之间差异,并据此判断先后顺序。
```java
import java.time.Duration;
import java.time.Instant;
public class CompareWithInstantAndDuration {
public static void main(String[] args) {
String instantStr1 = "2023-09-15T14:48:00Z";
String instantStr2 = "2023-09-16T14:48:00Z";
Instant inst1 = Instant.parse(instantStr1);
Instant inst2 = Instant.parse(instantStr2);
Duration durationBetween = Duration.between(inst1, inst2);
boolean isFirstEarlierThanSecond = !durationBetween.isNegative();
if(isFirstEarlierThanSecond && !durationBetween.isZero()){
System.out.println("instantStr1 occurs before instantStr2 by "+durationBetween.toDays()+" days.");
} else if(durationBetween.isZero()){
System.out.println("instants refer to exactly the same moments.");
} else{
System.out.println("instantStr1 actually comes after instantStr2!");
}
}
}
```
这种方式不仅限定了输入必须遵循 ISO 8601 的标准格式化规则,而且提供了更加直观的结果展示[^5]。
阅读全文
相关推荐
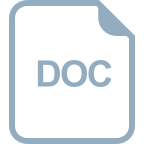
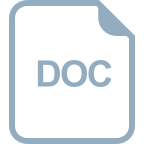
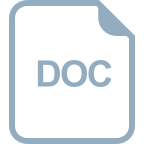


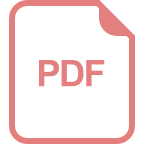







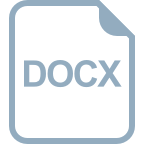




