java五子棋小游戏人机对战
时间: 2024-12-25 20:19:14 浏览: 16
### 使用Java实现五子棋小游戏人机对战
#### 游戏框架搭建
为了创建一个完整的五子棋游戏,首先需要构建基本的游戏框架。这包括初始化棋盘、处理玩家输入以及显示当前状态。
```java
public class GomokuGame {
private static final int SIZE = 15;
private char[][] board;
public GomokuGame() {
board = new char[SIZE][SIZE];
initializeBoard();
}
private void initializeBoard() {
for (int i = 0; i < SIZE; ++i) {
Arrays.fill(board[i], '.');
}
}
// 打印棋盘方法
public void printBoard() {
System.out.println(" a b c d e f g h i j k l m n o");
for (int row = 0; row < SIZE; ++row) {
System.out.print((char)(row + 'A') + " ");
for (int col = 0; col < SIZE; ++col) {
System.out.print(board[row][col] + " ");
}
System.out.println();
}
}
}
```
此部分代码定义了`GomokuGame`类并设置了初始条件[^1]。
#### 用户交互与AI逻辑
为了让计算机能够参与其中作为对手,需加入简单的AI模块。此处采用基于权值评估的方法决定最佳走法:
```java
import java.util.Random;
class AIPlayer {
private static final Random randomGenerator = new Random();
/**
* 计算每个格子的重要性得分,并返回最高分对应的坐标。
*/
Point findBestMove(char[][] board, boolean isMaximizingPlayer) {
double bestValue = Double.NEGATIVE_INFINITY;
Point move = null;
for (int y = 0; y < board.length; ++y) {
for (int x = 0; x < board[y].length; ++x) {
if (board[y][x] == '.') { // 如果该位置为空,则尝试放置棋子并计算其价值
double currentValue = evaluatePosition(x, y, board.clone(), !isMaximizingPlayer);
if (currentValue > bestValue || (currentValue == bestValue && randomGenerator.nextBoolean())) {
bestValue = currentValue;
move = new Point(x, y);
}
}
}
}
return move;
}
private double evaluatePosition(int posX, int posY, char[][] tempBoard, boolean maximizingPlayer) {
// 这里应该编写具体的评分函数...
return Math.random(); // 随机数仅用于示例目的
}
}
// 辅助类表示二维平面上的一个点
record Point(int x, int y) {}
```
上述实现了基础版本的AI决策流程,实际应用中应替换随机打分为具体策略分析[^4]。
#### 图形界面开发
考虑到用户体验,可以利用图形库如JavaFX来增强视觉效果。通过绑定事件处理器响应用户的点击动作,在界面上动态更新棋局进展。
```xml
<!-- FXML 文件片段 -->
<GridPane fx:id="gameBoard" alignment="CENTER">
<!-- 动态加载棋盘单元格... -->
</GridPane>
```
```java
@FXML
private GridPane gameBoard;
@Override
public void initialize(URL location, ResourceBundle resources) {
setupChessBoardUI(gameBoard);
gameBoard.setOnMouseClicked(event -> handleUserInput(event));
}
private void setupChessBoardUI(GridPane pane) {
// 构建GUI上的棋盘结构...
}
@FXML
void handleUserInput(MouseEvent event) {
Node node = ((Node)event.getSource());
Integer column = GridPane.getColumnIndex(node);
Integer row = GridPane.getRowIndex(node);
makeMove(row.intValue(), column.intValue());
}
```
这段展示了如何设置鼠标监听器捕捉用户操作,并据此触发相应的业务逻辑处理[^3]。
阅读全文
相关推荐




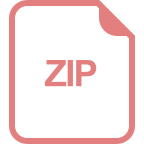
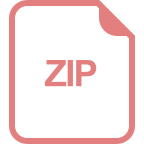










