Failed to obtain JDBC Connection; nested exception is java.sql.SQLException: com.cloudera.impala.jdbc.Driver
时间: 2023-11-24 09:53:02 浏览: 125
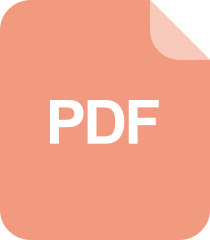
解决IDEA错误 Cause: java.sql.SQLException: The server time zone value的问题
根据提供的引用内容,这个错误信息表明在尝试建立JDBC连接时出现了问题。具体来说,它指出了一个嵌套的SQLException,该异常的原因是无法找到名为“com.cloudera.impala.jdbc.Driver”的驱动程序。这通常是由于缺少相应的JDBC驱动程序或驱动程序未正确配置所致。
解决此问题的步骤如下:
1. 确保已正确安装并配置了所需的JDBC驱动程序。在这种情况下,需要确保已正确安装Cloudera Impala JDBC驱动程序。
2. 确保在代码中正确指定了JDBC驱动程序的位置。这通常涉及到在代码中指定正确的JDBC URL和驱动程序类名。
3. 确保数据库服务器正在运行,并且可以从您的应用程序服务器上的网络访问该服务器。
以下是一个Java代码示例,演示如何建立JDBC连接并执行查询:
```java
import java.sql.*;
public class JdbcExample {
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// Register JDBC driver
Class.forName("com.cloudera.impala.jdbc.Driver");
// Open a connection
System.out.println("Connecting to database...");
conn = DriverManager.getConnection("jdbc:impala://localhost:21050/default", "<username>", "<password>");
// Execute a query
System.out.println("Creating statement...");
stmt = conn.createStatement();
String sql;
sql = "SELECT id, name, age FROM employees";
rs = stmt.executeQuery(sql);
// Extract data from result set
while (rs.next()) {
// Retrieve by column name
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
// Display values
System.out.print("ID: " + id);
System.out.print(", Name: " + name);
System.out.println(", Age: " + age);
}
// Clean-up environment
rs.close();
stmt.close();
conn.close();
} catch (SQLException se) {
// Handle errors for JDBC
se.printStackTrace();
} catch (Exception e) {
// Handle errors for Class.forName
e.printStackTrace();
} finally {
// Finally block used to close resources
try {
if (stmt != null)
stmt.close();
} catch (SQLException se2) {
} // nothing we can do
try {
if (conn != null)
conn.close();
} catch (SQLException se) {
se.printStackTrace();
} // end finally try
} // end try
}
}
```
阅读全文
相关推荐
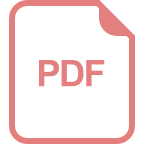
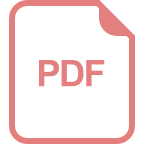















