如何获取C语言中实现文件路径遍历(mid函数)的最低级(底层)代码,以实现100%覆盖率的文件操作?
时间: 2024-11-12 12:37:27 浏览: 10
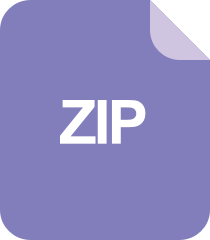
二叉树的创建与遍历C语言实现代码.zip
在C语言中,实现文件路径遍历通常涉及到系统调用,尤其是当你需要处理目录结构时。由于C标准库提供的函数如`opendir()`, `readdir()`, 和 `closedir()` 可以完成基本的目录遍历,但它们并不涉及底层的文件系统操作,因为这些都是操作系统层面的功能。
为了实现完全底层的文件路径遍历,你需要直接访问文件描述符(file descriptor)或者通过FUSE(Filesystem in Userspace)这样的用户空间文件系统框架。以下是一个简单的示例,展示了如何使用`open()`, `readlink()`, 和`lstat()`等系统调用来遍历目录:
```c
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
#include <dirent.h>
#include <stdio.h>
#include <errno.h>
#define MAX_PATH_LEN 4096
// 获取文件描述符并检查是否为目录
int open_dir(const char* path) {
int fd = open(path, O_RDONLY);
if (fd == -1) {
perror("Error opening directory");
return -1;
}
struct stat sb;
if (fstat(fd, &sb) != 0) {
perror("Error fstatting directory");
close(fd);
return -1;
}
// 检查是否是目录
if (!S_ISDIR(sb.st_mode)) {
printf("%s is not a directory.\n", path);
close(fd);
return -1;
}
return fd;
}
// 遍历目录内容
void traverse_directory(int fd) {
char link_path[MAX_PATH_LEN];
struct dirent *entry;
while ((entry = readdir(fd))) {
if (entry->d_type == DT_DIR) { // 如果是目录
link_path[0] = '\0';
ssize_t len = readlink(entry->d_name, link_path, sizeof(link_path) - 1);
if (len > 0 && len < MAX_PATH_LEN && link_path[len - 1] == '\0') {
printf("%s/\n", entry->d_name);
traverse_directory(open_dir(link_path));
} else {
printf("%s is a broken symlink.\n", entry->d_name);
}
} else { // 如果是文件
printf("%s\n", entry->d_name);
}
}
closedir(fd);
}
int main() {
int dir_fd = open_dir("/path/to/directory");
if (dir_fd >= 0) {
traverse_directory(dir_fd);
close(dir_fd);
}
return 0;
}
```
这个示例假设你可以读取链接到其他目录的链接,并且忽略了权限错误。然而,请注意,这仅是基础演示,实际应用中可能会遇到更复杂的情况,比如递归权限、硬链接处理以及跨分区的文件系统导航。此外,对于100%覆盖率的文件操作测试,你需要编写额外的测试用例以涵盖所有可能的边缘情况和异常处理。
阅读全文
相关推荐
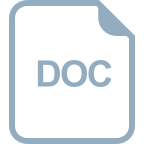
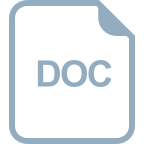
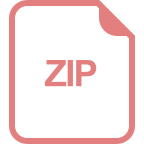
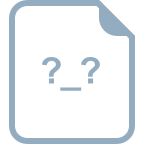
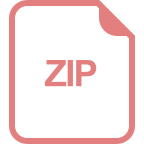
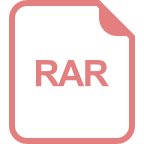
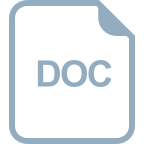
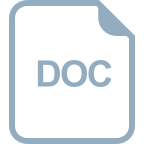
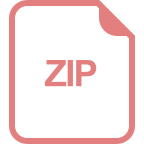
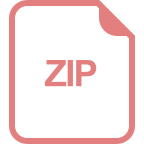
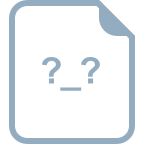
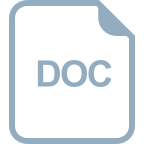
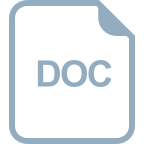
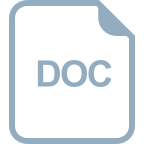