编写C语言函数,根据所给日期求从公元纪年开始到该日期的总天数。
时间: 2024-10-14 20:16:11 浏览: 6
在C语言中编写一个计算特定日期距离公元元年天数的函数,通常会涉及到闰年的判断以及月份天数的不同。以下是一个简单的示例,这个函数假设输入的是公历日期(即格里高利历),并且不处理公元前的日期:
```c
#include <stdio.h>
int days_from_era(int year, int month, int day) {
// 0 表示公元纪年
if (year < 1)
return -1; // 无效的公元前日期
int total_days = 0;
for (int y = 1; y < year; y++) { // 从公元1年到输入的年份
// 每四年一闰,百年不闰,四百年再闰(也就是闰年规则)
if ((y % 4 == 0 && y % 100 != 0) || (y % 400 == 0)) {
total_days += 366; // 平年365,闰年366
} else {
total_days += 365; // 非闰年
}
}
// 计算输入月份之前的天数(包括当前月的前几个月)
switch(month) {
case 1: case 2:
total_days += day + (month == 2 && is_leap_year(year));
break;
default:
total_days += day - 1; // 从3月开始,每个月减去1天
for (int m = 3; m < month; m++)
total_days += days_in_month(m, year);
break;
}
return total_days;
}
// 辅助函数检查是否是闰年
bool is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
// 获取给定月份的天数
int days_in_month(int month, int year) {
static const int days_in_month[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
return days_in_month[month - 1];
}
// 测试函数
int main() {
int year, month, day;
printf("请输入年、月、日(例如:2023 3 15): ");
scanf("%d %d %d", &year, &month, &day);
int total_days = days_from_era(year, month, day);
printf("从公元纪年起至给定日期共有 %d 天\n", total_days);
return 0;
}
```
请注意,这个函数仅适用于公历,并且不考虑儒略历和伊斯兰历等其他历法。此外,上述代码简化了对闰年的判断,实际应用中可能需要更精确的算法。
相关推荐
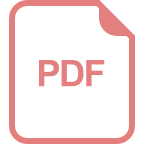
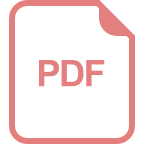
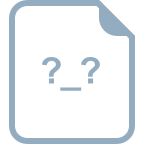














