matlab实现刀具半径补偿
时间: 2024-06-23 11:03:03 浏览: 11
在MATLAB中,刀具半径补偿(Tool Radius Compensation, TRC)是一种用于补偿加工过程中的刀具几何形状的方法,特别是在轮廓加工时,由于刀具的实际路径可能受刀具尺寸影响而不同于理想路径。以下是在MATLAB中实现刀具半径补偿的基本步骤:
1. **理解工具数据**:
首先,你需要知道刀具的半径和偏移方向,这些信息通常作为参数存储在加工程序中。
2. **设置坐标系**:
在编程时,你需要将机床的工作坐标系(G54-G59或G92)转换为一个带补偿的局部坐标系。这样,当执行带有补偿的G代码时,实际的刀具位置会在原始位置基础上加上补偿。
3. **使用G-code指令**:
使用特定的G-code指令来启用和配置刀具半径补偿。例如:
```
% 启用刀具半径左补偿
G41;
% 关闭补偿
G40;
```
对于不同类型的补偿(左补偿、右补偿),选择合适的指令。
4. **编写补偿算法**:
如果需要自定义补偿计算,可以编写循环,对每一个加工点应用刀具半径补偿。这通常涉及到向切线方向移动一个等于刀具半径的偏移量。
5. **测试和验证**:
完成程序后,你需要在仿真环境中(如Simulink或MATLAB的 Simscape Multibody)进行测试,确保补偿后的轨迹正确无误。
6. **保存和加载程序**:
将TRC代码保存为单独的模块或函数,以便在需要的时候重复调用。
相关问题
matlab实现盲元补偿
盲元补偿是一种用于去除信号中的系统失真的方法,但是在没有先验信息的情况下,这个过程是盲目的。Matlab提供了一些工具和函数来实现盲元补偿。
下面是一个使用Matlab实现盲元补偿的简单步骤:
1. 读入待处理的信号
可以使用Matlab中的`audioread`函数读入音频信号。
```matlab
[x,fs]=audioread('test.wav');
```
2. 构建盲源模型
盲源模型是指原始信号与目标信号之间的关系,可以使用ICA(独立成分分析)或者PCA(主成分分析)等方法进行构建。
以ICA为例,使用Matlab中的`fastICA`函数可以进行盲源分离。
```matlab
[S,A,W]=fastica(x');
```
其中,`S`是分离后的信号矩阵,`A`是混合矩阵,`W`是逆混合矩阵。
3. 提取混叠矩阵
将目标信号与混叠矩阵相乘可以得到混叠后的信号,因此需要提取混叠矩阵。
```matlab
H=A\eye(size(A));
```
其中,`\`表示矩阵的逆。
4. 进行盲元补偿
使用混叠矩阵进行盲元补偿。
```matlab
y=x*H';
```
5. 输出结果
将处理后的信号写入到一个新的音频文件中。
```matlab
audiowrite('result.wav',y,fs);
```
以上就是使用Matlab实现盲元补偿的简单步骤,具体的实现方法可以根据实际情况进行调整和优化。
matlab实现色散补偿
Matlab可以用于实现色散补偿,以下是一种常见的方法:
1. 首先,你需要获取原始的光学信号数据。这可以是从实验中测量得到的数据,或者是从模拟器或其他来源获取的数据。
2. 接下来,你需要对原始数据进行预处理,包括去噪和滤波等操作。这可以通过使用Matlab中的信号处理工具箱来实现。
3. 然后,你需要确定色散补偿算法。常见的色散补偿算法包括基于时域的算法和频域的算法。其中,频域算法更常用,因为它们可以更好地处理频率分量。
4. 在Matlab中,你可以使用FFT(快速傅里叶变换)函数将信号从时域转换到频域。然后,你可以应用色散补偿算法来调整频域中的相位和幅度。
5. 完成色散补偿后,你可以使用IFFT(逆傅里叶变换)函数将信号从频域转换回时域。
6. 最后,你可以对补偿后的信号进行进一步的分析和处理,以满足你的特定需求。
相关推荐
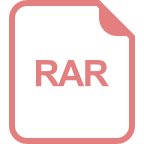












