java6、对象数组输入多个学生的学号,姓名,数学,语文,英语成绩,按总分从大到小排序。
时间: 2024-10-26 17:04:08 浏览: 20
在Java 6中,你可以使用ArrayList存储学生对象,并结合Collections.sort()函数对学生成绩列表进行排序。首先,你需要创建一个`Student`类,包含学号、姓名以及各科成绩属性,然后实现Comparable接口以提供自然排序的方式:
```java
// 定义 Student 类
class Student implements Comparable<Student> {
private int studentId;
private String name;
private double mathScore;
private double chinesesScore;
private double englishScore;
// 构造函数、getter和setter方法
@Override
public int compareTo(Student other) {
return Double.compare(this.getTotalScore(), other.getTotalScore());
}
private double getTotalScore() {
return mathScore + chinesesScore + englishScore;
}
}
public class Main {
public static void main(String[] args) {
// 创建 ArrayList 存储学生对象
List<Student> students = new ArrayList<>();
// 输入多个学生数据
for (int i = 0; i < numStudents; i++) { // 假设 numStudents 是你想要输入的学生数量
Scanner scanner = new Scanner(System.in);
System.out.println("请输入第 " + (i+1) + " 位学生的学号、姓名和各科成绩:");
int id = scanner.nextInt();
String name = scanner.next();
double math = scanner.nextDouble();
double chinese = scanner.nextDouble();
double english = scanner.nextDouble();
Student student = new Student();
student.setStudentId(id);
student.setName(name);
student.setMathScore(math);
student.setChinesesScore(chinese);
student.setEnglishScore(english);
students.add(student);
}
// 按照总分从高到低排序
Collections.sort(students);
// 输出排序后的学生信息
for (Student student : students) {
System.out.println("学生ID:" + student.getStudentId() +
", 姓名:" + student.getName() +
", 总分:" + student.getTotalScore());
}
}
}
```
在这个示例中,用户会被提示输入每个学生的数据,然后程序会按照总分降序排列并打印出来。
阅读全文
相关推荐
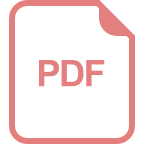
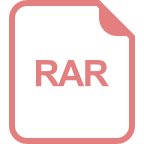
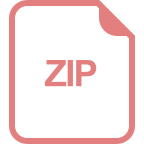
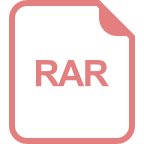
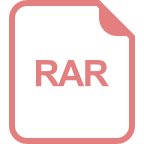
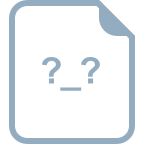
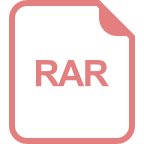
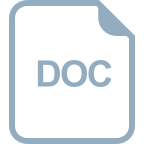
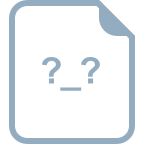
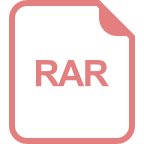
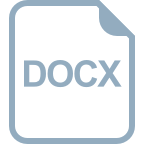
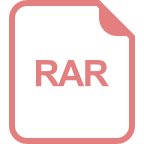
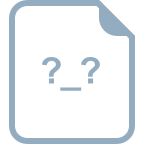
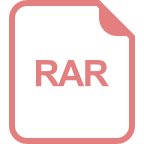
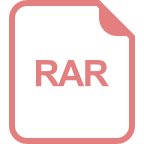
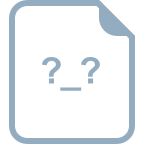
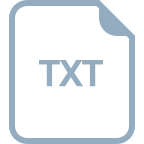
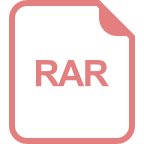