springboot操作redis 怎么操作redis中的不同数据库
时间: 2023-07-29 11:09:56 浏览: 165
在Spring Boot中操作Redis的不同数据库,可以通过配置RedisTemplate来实现。下面是一个示例:
首先,需要在application.properties(或application.yml)文件中配置Redis连接信息和数据库索引:
```
spring.redis.host=127.0.0.1
spring.redis.port=6379
spring.redis.database=0 # 默认数据库索引为0,可以根据需要修改
```
接下来,创建一个RedisConfig类来配置RedisTemplate:
```java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.connection.RedisStandaloneConfiguration;
import org.springframework.data.redis.connection.lettuce.LettuceConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.GenericJackson2JsonRedisSerializer;
import org.springframework.data.redis.serializer.StringRedisSerializer;
@Configuration
public class RedisConfig {
@Bean
public RedisConnectionFactory redisConnectionFactory() {
RedisStandaloneConfiguration config = new RedisStandaloneConfiguration();
config.setHostName("127.0.0.1");
config.setPort(6379);
config.setDatabase(1); // 设置数据库索引为1
return new LettuceConnectionFactory(config);
}
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(redisConnectionFactory);
template.setKeySerializer(new StringRedisSerializer());
template.setValueSerializer(new GenericJackson2JsonRedisSerializer());
template.setHashKeySerializer(new StringRedisSerializer());
template.setHashValueSerializer(new GenericJackson2JsonRedisSerializer());
return template;
}
}
```
在上面的示例中,我们通过RedisStandaloneConfiguration设置了连接信息和数据库索引,其中数据库索引设置为1。然后,我们使用LettuceConnectionFactory创建Redis连接工厂。最后,我们配置了RedisTemplate,设置了序列化器和连接工厂。
现在,你可以在需要操作Redis的地方注入RedisTemplate,并使用它来操作不同的数据库了。例如:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.stereotype.Service;
@Service
public class RedisService {
private final RedisTemplate<String, Object> redisTemplate;
@Autowired
public RedisService(RedisTemplate<String, Object> redisTemplate) {
this.redisTemplate = redisTemplate;
}
public void set(String key, Object value) {
redisTemplate.opsForValue().set(key, value);
}
public Object get(String key) {
return redisTemplate.opsForValue().get(key);
}
}
```
在上面的示例中,我们通过构造函数注入了RedisTemplate,并使用opsForValue()方法来操作键值对数据。
需要注意的是,如果使用不同的数据库索引,需要在RedisConfig类中对应的地方修改数据库索引值,例如config.setDatabase(1)表示使用数据库索引为1的数据库。
相关推荐
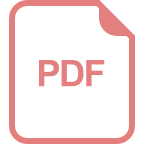














