实现线性表的顺序存储定义,完成顺序表的创建,删除,插入,查找等操作,完成两个有序线性表的合并,要求同样的数据元素只出现一次
时间: 2024-11-09 09:24:21 浏览: 32
实现线性表的顺序存储通常涉及到数组作为底层的数据结构,每个元素存储在一个连续的内存空间内。下面是基本的操作步骤和函数定义:
1. **顺序表定义**:
```c++
class SequentialList {
private:
int* data; // 存储元素的指针
int size; // 当前元素的数量
int capacity; // 底层数组的容量
public:
SequentialList(int initialCapacity); // 构造函数,初始化容量
~SequentialList(); // 析构函数,释放内存
// 其他操作
void insert(int value);
void deleteElement(int index);
int search(int target);
void merge(SequentialList& other); // 合并两个有序列表
bool isUnique(int value) const; // 检查值是否唯一
};
```
2. **创建顺序表**:
```c++
SequentialList::SequentialList(int initialCapacity) {
data = new int[initialCapacity];
capacity = initialCapacity;
size = 0;
}
```
3. **删除元素**:
```c++
void SequentialList::deleteElement(int index) {
if (index < 0 || index >= size) {
return;
}
memmove(data + index, data + index + 1, sizeof(int) * (size - index - 1));
size--;
if (size == capacity / 4 && capacity > 0) { // 如果还有大量空间,则扩容
resize(capacity * 2);
}
}
```
4. **插入元素**:
```c++
void SequentialList::insert(int value) {
if (size == capacity) {
resize(capacity * 2); // 扩容
}
data[size++] = value;
}
```
5. **查找元素**:
```c++
int SequentialList::search(int target) {
for (int i = 0; i < size; ++i) {
if (data[i] == target) {
return i;
}
}
return -1; // 未找到目标值
}
```
6. **合并有序列表**:
```c++
void SequentialList::merge(SequentialList& other) {
// 使用双指针法合并,检查当前元素是否小于其他列表的第一个元素
int* thisPtr = data + size - 1, *otherPtr = other.data + other.size - 1;
while (thisPtr >= data && otherPtr >= other.data) {
if (*thisPtr <= *otherPtr) {
data[--size] = *thisPtr;
thisPtr--;
} else {
data[--size] = *otherPtr;
otherPtr--;
}
}
// 将剩余的元素添加到此列表
if (otherPtr >= other.data) {
memcpy(data + size, other.data, sizeof(int) * (other.size - 1));
} else {
memcpy(data + size, thisPtr, sizeof(int) * (size - (thisPtr - data)));
memcpy(data + size, otherPtr, sizeof(int) * (other.size - (otherPtr - other.data)));
}
}
```
7. **检查唯一性**:
```c++
bool SequentialList::isUnique(int value) const {
for (int i = 0; i < size - 1; ++i) {
if (data[i] == value && data[i + 1] == value) {
return false;
}
}
return true;
}
```
注意:以上代码是一个简化的示例,实际实现可能需要考虑边界条件、异常处理以及更高效的数据移动方法。同时,这个版本假设了`resize()` 函数能安全地扩展数组。
阅读全文
相关推荐
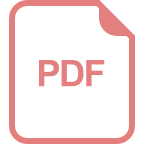
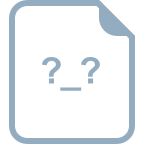
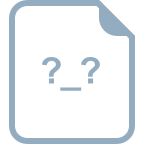
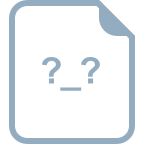
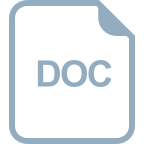



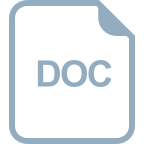
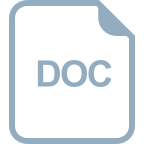
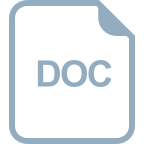
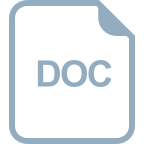
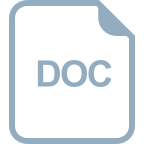



