python 解压缩zip文件
时间: 2024-03-19 09:38:02 浏览: 20
在Python中解压缩zip文件可以使用zipfile模块。下面是一个简单的示例代码:
```python
import zipfile
def unzip_file(zip_path, extract_path):
with zipfile.ZipFile(zip_path, 'r') as zip_ref:
zip_ref.extractall(extract_path)
# 调用函数进行解压缩
unzip_file('example.zip', 'extracted_folder')
```
上述代码中,`zip_path`是要解压缩的zip文件路径,`extract_path`是解压缩后文件的保存路径。通过`zipfile.ZipFile`打开zip文件,然后使用`extractall`方法将文件解压缩到指定路径。
相关问题
python 解压缩 zip文件
Python提供了zipfile模块来进行ZIP文件的解压缩操作。下面是一个简单的例子,演示如何使用zipfile模块解压缩整个ZIP文件和逐个解压缩文件:
```python
import zipfile
# 解压缩整个ZIP文件
with zipfile.ZipFile('example.zip', 'r') as zip_ref:
zip_ref.extractall('example')
# 逐个解压缩文件
with zipfile.ZipFile('example.zip', 'r') as zip_ref:
for file in zip_ref.namelist():
zip_ref.extract(file, 'example')
```
在上面的例子中,我们首先使用`ZipFile`函数打开ZIP文件,然后使用`extractall`方法解压缩整个ZIP文件到指定目录。接着,我们使用`namelist`方法获取ZIP文件中所有文件的名称列表,然后使用`extract`方法逐个解压缩文件到指定目录。
python解压缩分卷zip文件
你可以使用`zipfile`模块来解压缩分卷的zip文件。下面是一个示例代码:
```python
import zipfile
def unzip_split_zip(filename):
# 获取分卷的zip文件名列表
filenames = [filename] # 添加第一个分卷的文件名
i = 1
while True:
part_filename = f"{filename}.z{i}"
if part_filename in zipfile.ZipFile.namelist(part_filename):
filenames.append(part_filename)
i += 1
else:
break
# 将所有分卷文件合并成一个完整的zip文件
with open(filename, 'wb') as output_file:
for part_filename in filenames:
with open(part_filename, 'rb') as part_file:
output_file.write(part_file.read())
# 解压缩合并后的zip文件
with zipfile.ZipFile(filename, 'r') as zip_ref:
zip_ref.extractall()
# 使用示例
unzip_split_zip('data.zip')
```
在这个示例中,我们首先将所有分卷的zip文件合并成一个完整的zip文件,然后再进行解压缩操作。你只需要将`unzip_split_zip`函数的参数替换为你要解压缩的分卷zip文件的文件名。
相关推荐
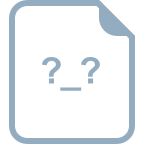












