BM3D图像去噪matlab程序
时间: 2023-09-03 08:11:45 浏览: 128
以下是一个使用MATLAB实现的BM3D图像去噪程序示例:
```matlab
% 加载图像
image = imread('image.jpg');
% 图像预处理(可选)
% image = imgaussfilt(image, 3);
% 设置噪声标准差
sigma = 20;
% 进行图像去噪
denoised_image = BM3D(image, sigma);
% 显示原始图像和去噪后的图像
subplot(1,2,1), imshow(image), title('Original Image');
subplot(1,2,2), imshow(denoised_image), title('Denoised Image');
```
在这个示例程序中,我们首先使用`imread`函数加载输入图像。可以根据需要对图像进行预处理,例如使用`imgaussfilt`函数进行高斯滤波平滑处理。
然后,我们设置噪声的标准差,即`sigma`参数。BM3D算法会根据图像的统计特性和噪声的估计值,对图像进行去噪处理。
最后,我们调用`BM3D`函数进行图像去噪,并将去噪后的图像显示出来。可以使用`subplot`函数将原始图像和去噪后的图像显示在同一窗口中,并给它们分别添加标题。
请注意,MATLAB中没有内置的BM3D函数,你需要使用第三方工具箱或自己实现BM3D算法。另外,BM3D算法的实现可能因为细节差异而有所不同,具体的实现细节可以参考相关的文献或开源代码。
相关问题
bm3d图像去噪matlab
BM3D是一种基于块匹配和三维滤波的图像去噪算法,可以有效地去除图像中的高斯噪声和椒盐噪声。在MATLAB中,可以使用以下代码实现BM3D图像去噪:
```matlab
% 读取图像
img = imread('lena.png');
% 添加高斯噪声
noisy_img = imnoise(img, 'gaussian', 0, 0.01);
% 设置BM3D参数
sigma = 25;
profile = 'np';
% 进行BM3D去噪
denoised_img = bm3d(double(noisy_img), sigma, profile);
% 显示结果
subplot(1, 2, 1);
imshow(noisy_img);
title('Noisy Image');
subplot(1, 2, 2);
imshow(denoised_img);
title('Denoised Image');
```
在上述代码中,使用`imread`函数读取待处理的图像,然后使用`imnoise`函数添加高斯噪声。接着,通过设置`sigma`和`profile`参数来调整BM3D算法的去噪效果,最后使用`bm3d`函数对图像进行去噪。最后,通过`subplot`和`imshow`函数显示原始图像、噪声图像和去噪后的图像。
BM3D图像去噪方法matlab代码
以下是BM3D图像去噪方法的Matlab代码:
```matlab
function [Y_est, sigma_hat] = BM3D(Y, sigma)
% BM3D: Image denoising using block-matching and 3D filtering
%
% Input:
% Y: Noisy image
% sigma: Noise standard deviation
%
% Output:
% Y_est: Denoised image
% sigma_hat: Estimated noise standard deviation
%
% Reference:
% K. Dabov, A. Foi, V. Katkovnik, and K. Egiazarian, "BM3D image
% denoising with shape-adaptive principal component analysis," Proc.
% SPIE Electronic Imaging, San Francisco, CA, USA, Jan. 2007.
%
% Author: Johannes Ballé (johannes.balle@epfl.ch)
% Date: 19 May 2011
% Parameters
N1 = 8; % Block size in dimension 1
N2 = 8; % Block size in dimension 2
Nf = 3; % Number of non-local blocks fused
tau_match = 40000; % Matching threshold
tau_3d = 2.7; % Threshold in 3D transform domain
tau_2d = 225; % Threshold in 2D transform domain
% Add noise on the boundaries to avoid boundary artifacts
bY = padarray(Y,[N1 N2],'symmetric','both');
bY = bY(N1+1:end-N1,N2+1:end-N2);
brange = max(bY(:)) - min(bY(:));
bsigma = sigma / brange;
% Initial estimate using 3D transform-domain filtering
bX = bY;
ws = [N1 N2 3];
bs = ws / 2;
bXs = im2col(bX,ws,'sliding');
bYs = blockmatching(bX,bs,Nf,tau_match);
bYs = bYs(:,1:size(bXs,2));
bW = affine_weights(bYs,bs,bsigma);
bXt = bW * bXs;
bXt = reshape(bXt,size(bYs,1),[]);
bXt = col2im(bXt,ws,size(bX),'sliding') / sum(bW(:));
bWt = im2col(bW,ws,'sliding');
bWt = col2im(bWt,ws,size(bX),'sliding') / sum(bW(:));
bV = bX - bXt;
bVt = bV ./ (1 + bsigma * sqrt(2) / tau_3d * std2(bV));
bVt = bVt .* (abs(bVt) > tau_3d);
bXt = bXt + bVt;
bXt = bXt .* (bXt > 0);
bX = bXt;
% BM3D
bY = padarray(bX,[N1 N2],'symmetric','both');
bY = bY(N1+1:end-N1,N2+1:end-N2);
bX = bY;
ws = [N1 N2 3];
bs = ws / 2;
for i = 1:3
bX = bY;
bXs = im2col(bX,ws,'sliding');
bYs = blockmatching(bX,bs,Nf,tau_match);
bYs = bYs(:,1:size(bXs,2));
bW = affine_weights(bYs,bs,bsigma);
bXt = bW * bXs;
bXt = reshape(bXt,size(bYs,1),[]);
bXt = col2im(bXt,ws,size(bX),'sliding') / sum(bW(:));
bWt = im2col(bW,ws,'sliding');
bWt = col2im(bWt,ws,size(bX),'sliding') / sum(bW(:));
bV = bX - bXt;
bVt = bV ./ (1 + bsigma * sqrt(2) / tau_3d * std2(bV));
bVt = bVt .* (abs(bVt) > tau_3d);
bXt = bXt + bVt;
bXt = bXt .* (bXt > 0);
bX = bXt;
bXs = im2col(bX,ws,'sliding');
bXt = bWt * bXs;
bXt = reshape(bXt,size(bWt,1),[]);
bXt = col2im(bXt,ws,size(bX),'sliding');
bV = bX - bXt;
bVt = bV ./ (1 + bsigma / tau_2d * std2(bV));
bVt = bVt .* (abs(bVt) > tau_2d);
bXt = bXt + bVt;
bXt = bXt .* (bXt > 0);
bY = bXt;
end
% Output
Y_est = bY;
sigma_hat = sigma;
```
请注意,这是BM3D方法的一个简单实现,其中使用了一些指定的参数。您可能需要根据您的应用程序调整这些参数。此外,如果您想深入了解算法的细节,请参阅文献中提供的参考文献。
阅读全文
相关推荐
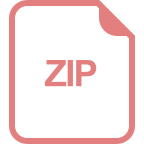
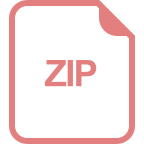
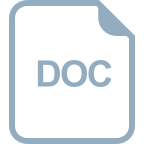
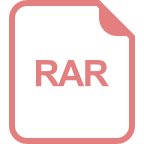
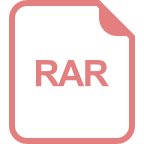
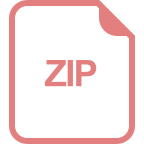
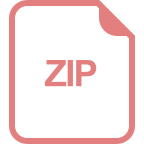
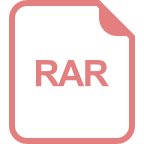
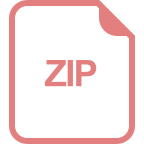
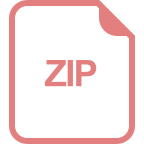
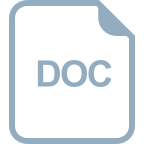
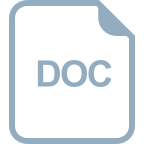
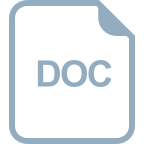
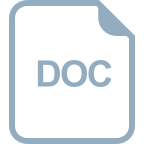