对学生成绩管理系统的代码c语言
时间: 2024-06-19 10:03:43 浏览: 99
学生成绩管理系统是一个常见的程序设计项目,主要包括学生信息录入、成绩录入、成绩查询、成绩排序等功能。以下是一个简单的学生成绩管理系统的C语言代码示例:
```c
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#define MAX_STU_NUM 100
typedef struct student {
int id; // 学号
char name[20]; // 姓名
float score; // 成绩
} Student;
int menu() {
int choice;
printf("\n\n");
printf("**********************************************\n");
printf("* *\n");
printf("* 学生成绩管理系统 *\n");
printf("* *\n");
printf("* 1. 录入学生信息 *\n");
printf("* 2. 录入学生成绩 *\n");
printf("* 3. 查询学生成绩 *\n");
printf("* 4. 按成绩排序 *\n");
printf("* 0. 退出程序 *\n");
printf("* *\n");
printf("**********************************************\n");
printf("请输入你的选择:");
scanf("%d", &choice);
return choice;
}
void input_student(Student *p) {
printf("请输入学生的学号:");
scanf("%d", &(p->id));
printf("请输入学生的姓名:");
scanf("%s", p->name);
}
void input_score(Student *p) {
printf("请输入学生的成绩:");
scanf("%f", &(p->score));
}
void print_student(Student *p) {
printf("%d\t%s\t%.2f\n", p->id, p->name, p->score);
}
void print_all_students(Student *students, int count) {
printf("学号\t姓名\t成绩\n");
for (int i = 0; i < count; i++) {
print_student(students + i);
}
}
void query_score(Student *students, int count) {
int id;
printf("请输入要查询成绩的学生学号:");
scanf("%d", &id);
for (int i = 0; i < count; i++) {
if (students[i].id == id) {
print_student(students + i);
return;
}
}
printf("找不到该学生的信息!\n");
}
void sort_by_score(Student *students, int count) {
for (int i = 0; i < count - 1; i++) {
for (int j = 0; j < count - i - 1; j++) {
if (students[j].score < students[j + 1].score) {
Student temp = students[j];
students[j] = students[j + 1];
students[j + 1] = temp;
}
}
}
}
int main() {
Student students[MAX_STU_NUM];
int count = 0;
while (1) {
int choice = menu();
switch (choice) {
case 0:
printf("再见!\n");
exit(0);
case 1:
input_student(students + count);
count++;
break;
case 2:
input_score(students + count - 1);
break;
case 3:
query_score(students, count);
break;
case 4:
sort_by_score(students, count);
print_all_students(students, count);
break;
default:
printf("输入错误,请重新输入!\n");
break;
}
}
}
```
以上代码实现了基本的学生成绩管理系统功能,包括录入学生信息、录入成绩、查询成绩和按成绩排序。你可以根据自己的需求对代码进行修改和扩展。
阅读全文
相关推荐


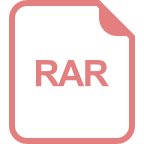
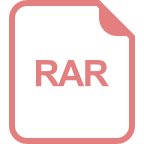



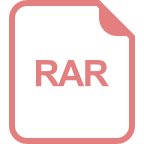
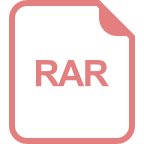

