反复读取文件夹中的每张图片的rgb信息,每张图片建立一个对应的txt来保存读取到的rgb信息,保存之前删除对应的txt文本内容。c++实现,使用dirent库遍历文件,不使用用图像处理库,要求可以在ubuntu上可以运行
时间: 2024-04-15 07:27:43 浏览: 74
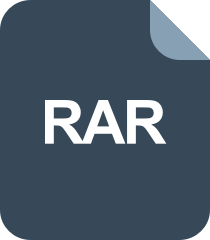
读取bmp图片文件,并获取任意一点的RGB值,并可按任意指定大小保存bmp文件

如果您希望在Ubuntu上使用dirent库来遍历文件夹并读取每张图片的RGB信息,并将其保存到对应的txt文件中,请参考以下示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <dirent.h>
void processImage(const std::string& imagePath) {
std::ifstream imageFile(imagePath, std::ios::binary);
if (!imageFile) {
std::cerr << "Failed to open image file: " << imagePath << std::endl;
return;
}
std::string txtPath = imagePath + ".txt";
std::ofstream txtFile(txtPath);
if (!txtFile) {
std::cerr << "Failed to create txt file: " << txtPath << std::endl;
return;
}
// Read the RGB values of each pixel
char buffer[3];
while (imageFile.read(buffer, sizeof(buffer))) {
unsigned char r = buffer[0];
unsigned char g = buffer[1];
unsigned char b = buffer[2];
// Write the RGB values to the txt file
txtFile << static_cast<int>(r) << " "
<< static_cast<int>(g) << " "
<< static_cast<int>(b) << "\n";
}
imageFile.close();
txtFile.close();
}
void clearTxtFiles(const std::string& folderPath) {
DIR* dir = opendir(folderPath.c_str());
if (dir == nullptr) {
std::cerr << "Failed to open directory: " << folderPath << std::endl;
return;
}
dirent* entry;
while ((entry = readdir(dir)) != nullptr) {
std::string fileName = entry->d_name;
if (fileName != "." && fileName != "..") {
std::string filePath = folderPath + "/" + fileName;
if (fileName.find(".txt") != std::string::npos) {
std::remove(filePath.c_str());
}
}
}
closedir(dir);
}
int main() {
std::string folderPath = "/path/to/your/folder";
// Clear existing txt files
clearTxtFiles(folderPath);
// Process each image file in the folder
DIR* dir = opendir(folderPath.c_str());
if (dir == nullptr) {
std::cerr << "Failed to open directory: " << folderPath << std::endl;
return 1;
}
dirent* entry;
while ((entry = readdir(dir)) != nullptr) {
std::string fileName = entry->d_name;
if (fileName != "." && fileName != "..") {
std::string filePath = folderPath + "/" + fileName;
if (fileName.find(".jpg") != std::string::npos || fileName.find(".png") != std::string::npos) {
processImage(filePath);
}
}
}
closedir(dir);
return 0;
}
```
请将 `/path/to/your/folder` 替换为您实际的文件夹路径。
这段代码会首先清除文件夹中已有的txt文件,然后使用dirent库遍历文件夹中的每个文件,筛选出图片文件(.jpg或.png),逐个处理并生成对应的txt文件。每个txt文件中会保存对应图片的RGB信息,每行表示一个像素点的RGB值。
请注意,dirent库是一个C库,因此代码中需要包含相关的头文件,并使用C的字符串处理函数。
阅读全文
相关推荐
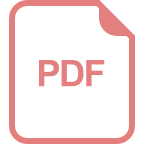
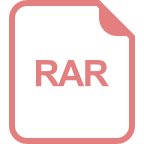
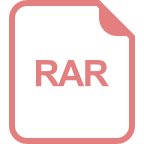
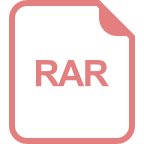
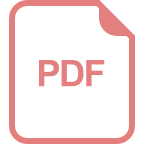
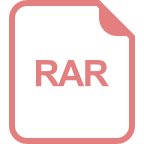
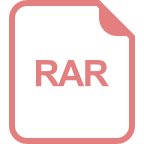
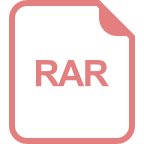
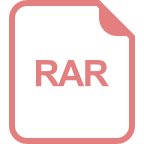
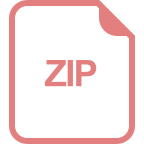
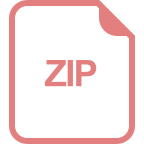
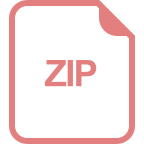
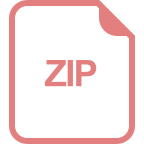
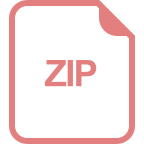