using System.Threading 中关于延迟执行下一条代码的写法
时间: 2024-12-15 12:16:56 浏览: 6
在`System.Threading`命名空间中,主要通过`Thread.Sleep`方法实现延迟执行下一条代码。不过,这种方法不是异步的,会导致主线程暂停,如果需要在其他线程上等待,可以使用`Timer`或者`Task.Delay`。以下是使用`Thread.Sleep`的例子:
```csharp
using System.Threading;
public void DelayExecution()
{
Console.WriteLine("开始执行...");
// 延迟1秒
Thread.Sleep(1000); // 1000毫秒等于1秒
Console.WriteLine("1秒后执行...");
}
```
在这个例子中,`Thread.Sleep`会让当前线程暂停执行指定的时间,然后恢复到下一行代码。
如果你想保持异步风格,可以在主线程上调用`Task.Delay`:
```csharp
using System.Threading.Tasks;
public async void AsyncDelayExecution()
{
Console.WriteLine("开始执行...");
await Task.Delay(1000); // 异步等待1秒
Console.WriteLine("1秒后执行...");
}
```
在这里,`await Task.Delay`会在延时期间释放主线程,让你的代码继续保持响应状态。
相关问题
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace WindowsFormsApp2 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void label1_Click(object sender, EventArgs e) { } private void button1_Click(object sender, EventArgs e) { label1.Text = "很好,天天向上"; } private void button2_Click(object sender, EventArgs e) { label1.Text = "想多了,还没睡醒吧!!!"; } private void button2_MouseEnter(object sender, EventArgs e) { Random r = new Random (); button2.Left = r.Next(this.ClientRectangle.Left, this.ClientRectangle.Width - button2.width); button2.Top = r.Next(this.clientRectangle.Top, this.ClientRectangle.Height - button2.Height);
我发现你在button2_MouseEnter方法中使用了小写的width和clientRectangle,这可能导致编译错误。正确的写法应该是大写字母的Width和ClientRectangle。此外,你还可以考虑添加一些边界检查,以确保button2不会超出窗口范围。例如,你可以使用Math.Max和Math.Min方法来限制button2的位置不超出窗口的左右和上下边界。
c#中有返回值的线程参数方法的写法
在 C# 中,可以使用委托和线程池来创建一个具有返回值的线程参数方法。以下是一个示例代码:
```
using System;
using System.Threading;
public class Program
{
public delegate int MyDelegate(string message);
static void Main(string[] args)
{
MyDelegate del = new MyDelegate(DoWork);
IAsyncResult result = del.BeginInvoke("Hello World", null, null);
// 主线程可以做其它事情
int returnValue = del.EndInvoke(result);
Console.WriteLine("返回值为:" + returnValue);
}
static int DoWork(string message)
{
Console.WriteLine("线程开始执行");
Thread.Sleep(3000);
Console.WriteLine("线程执行完毕");
return message.Length;
}
}
```
在上述代码中,首先定义了一个 `MyDelegate` 委托,它的参数是一个字符串,返回值是一个整数。然后在 `Main` 方法中,创建了一个委托实例 `del`,并使用 `BeginInvoke` 方法来异步启动一个线程执行 `DoWork` 方法。在主线程中可以做其它事情。最后使用 `EndInvoke` 方法来获取线程执行的返回值。
阅读全文
相关推荐
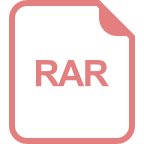
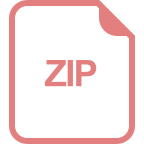
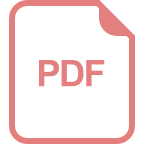
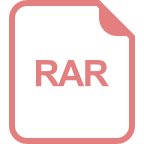
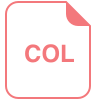

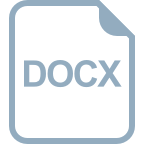
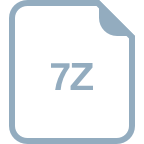
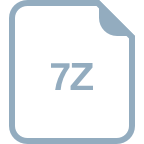
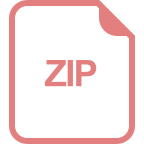
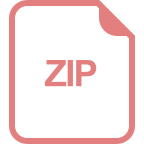
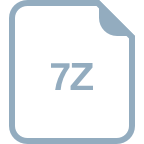