利用c语言实现时钟功能
时间: 2023-07-13 18:31:22 浏览: 88
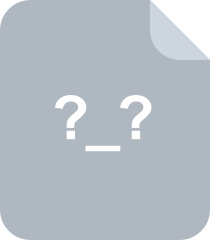
用c语言实现时钟
实现时钟功能可以通过获取当前时间并计算指针位置,然后在绘图界面上绘制时钟表盘、数字、指针等。具体实现步骤如下:
1. 引入 time.h 和 graphics.h 库文件;
2. 初始化绘图环境,使用 initgraph() 函数;
3. 定义变量存储当前时间,使用 time() 和 localtime() 函数获取本地时间,并使用结构体指针获取时、分、秒;
4. 计算指针在时钟表盘上的位置,可以使用三角函数来计算;
5. 绘制时钟表盘,包括圆形、刻度、数字等;
6. 绘制指针,使用 setlinestyle() 和 setcolor() 函数设置指针样式;
7. 在绘图界面上显示时钟,使用 outtextxy() 函数输出时间;
8. 关闭绘图环境,使用 closegraph() 函数。
下面是一个简单的示例代码:
```c
#include <time.h>
#include <graphics.h>
#include <math.h>
#define PI 3.1415926
int main()
{
int gd=DETECT,gm;
initgraph(&gd,&gm,""); // 初始化绘图环境
while(1) // 无限循环,不断更新时钟
{
time_t now;
struct tm *current;
char time_str[20];
int hour, minute, second;
int cx=320, cy=240; // 设置时钟中心点坐标
int r=200; // 设置时钟半径
// 获取当前时间
time(&now);
current=localtime(&now);
hour=current->tm_hour;
minute=current->tm_min;
second=current->tm_sec;
// 计算时针、分针、秒针在时钟表盘上的位置
int hx=cx+r/2*sin(2*PI/12*(hour%12)+2*PI/720*minute);
int hy=cy-r/2*cos(2*PI/12*(hour%12)+2*PI/720*minute);
int mx=cx+r/1.5*sin(2*PI/60*minute);
int my=cy-r/1.5*cos(2*PI/60*minute);
int sx=cx+r*sin(2*PI/60*second);
int sy=cy-r*cos(2*PI/60*second);
// 绘制时钟表盘
setcolor(BLACK);
setfillstyle(SOLID_FILL, WHITE);
circle(cx, cy, r);
floodfill(cx, cy, BLACK);
// 绘制刻度
for(int i=0; i<12; i++)
{
int x1=cx+(r-20)*sin(2*PI/12*i);
int y1=cy-(r-20)*cos(2*PI/12*i);
int x2=cx+r*sin(2*PI/12*i);
int y2=cy-r*cos(2*PI/12*i);
line(x1, y1, x2, y2);
}
// 绘制数字
char str[3];
for(int i=1; i<=12; i++)
{
sprintf(str, "%d", i);
int x=cx+(r-40)*sin(2*PI/12*i);
int y=cy-(r-40)*cos(2*PI/12*i);
outtextxy(x-8, y-8, str);
}
// 绘制指针
setlinestyle(SOLID_LINE, 2, 2);
setcolor(BLACK);
line(cx, cy, hx, hy);
setlinestyle(SOLID_LINE, 1, 2);
setcolor(BLACK);
line(cx, cy, mx, my);
setlinestyle(SOLID_LINE, 1, 1);
setcolor(RED);
line(cx, cy, sx, sy);
// 输出时间
sprintf(time_str, "%02d:%02d:%02d", hour, minute, second);
setcolor(BLACK);
outtextxy(280, 50, time_str);
delay(1000); // 延时1秒,更新时钟
cleardevice(); // 清空绘图区域
}
closegraph(); // 关闭绘图环境
return 0;
}
```
这段代码使用了 while 循环,不断更新时钟,使用 delay() 函数控制更新频率,使用 cleardevice() 函数清空绘图区域。在绘制时钟表盘、数字、指针等时,可以根据需要调整参数,使其更符合实际需求。
阅读全文
相关推荐
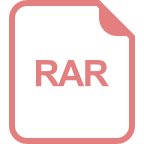
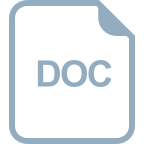
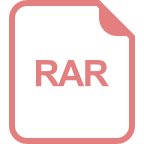
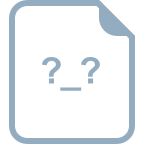
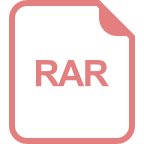
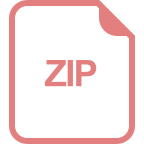
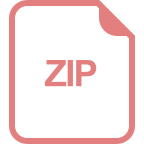
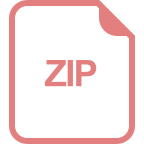
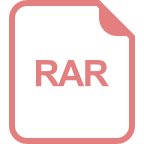
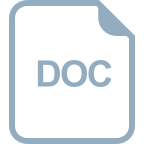
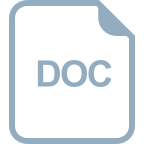
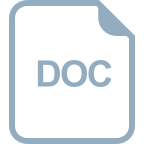
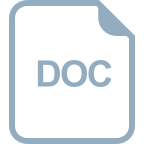
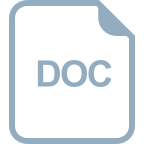
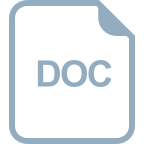
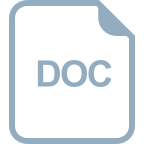