编写一个算法,利用栈的基本运算将定栈中的内容进逆转
时间: 2024-10-09 19:04:01 浏览: 34
要使用栈(Stack)的特性来反转其内容,我们可以创建一个新的栈,然后逐个弹出原栈的元素并压入新栈中。这种方法叫做“迭代法”,因为不需要用到递归或额外的数据结构。
以下是一个简单的C语言示例,展示了如何完成这个操作:
```c
#include <stdio.h>
#include <stdlib.h>
// 假设我们有一个定义好的栈类型
typedef struct Node {
int data;
struct Node* next;
} StackNode;
// 创建一个新的空栈
StackNode* createStack() {
StackNode* stack = (StackNode*)malloc(sizeof(StackNode));
if (!stack) {
printf("Memory allocation failed.\n");
return NULL;
}
stack->next = NULL;
return stack;
}
// 栈顶元素弹出并返回
int pop(StackNode** top) {
if (*top == NULL) {
printf("Stack is empty.\n");
return -1;
}
int value = (*top)->data;
StackNode* temp = *top;
*top = (*top)->next;
free(temp);
return value;
}
// 将一个值压入栈
void push(StackNode** top, int value) {
StackNode* newNode = (StackNode*)malloc(sizeof(StackNode));
if (!newNode) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
newNode->next = *top;
*top = newNode;
}
// 主函数,用于测试
void reverseStack(StackNode** top) {
StackNode* newTop = createStack();
while (!(*top)->next == NULL) {
push(newTop, pop(top));
} // 处理栈底元素
push(newTop, pop(top)); // 如果原栈不为空,添加栈底元素
// 显示反转后的栈
StackNode* current = newTop;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
// 测试
int main() {
StackNode* stack = createStack();
// 入栈操作,这里省略具体代码,假设已经入了一些元素
// ...
reverseStack(&stack);
return 0;
}
```
阅读全文
相关推荐
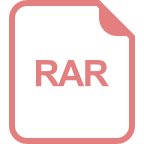
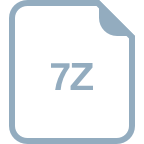
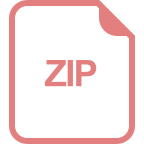










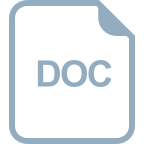
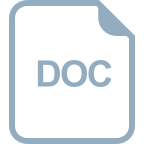
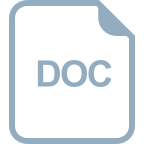
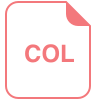
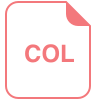
