C语言编写函数,去掉字符串末尾的空格。编写主函数,输入中间和末尾可能带一个或多个空格的字符串,显示该字符串,然后调用函数去掉末尾的字符串,再在主函数中显示去掉末尾空格的字符串。 输入:字符串,中间和末尾
时间: 2024-05-11 10:14:00 浏览: 40
可能带一个或多个空格
输出:显示原字符串,去掉末尾空格的字符串
```c
#include <stdio.h>
#include <string.h>
void removeSpace(char *str) {
int len = strlen(str);
while (len > 0 && str[len - 1] == ' ') {
str[len - 1] = '\0';
len--;
}
}
int main() {
char str[100];
printf("请输入字符串:");
fgets(str, sizeof(str), stdin);
printf("原字符串是:%s", str);
removeSpace(str);
printf("去掉末尾空格的字符串是:%s", str);
return 0;
}
```
相关问题
编写C语言函数将两个字符串用空格连接起来,要求在主函数里进行输入输出字符串。
好的,这个问题很简单。以下是C语言函数的代码示例:
```c
#include <stdio.h>
#include <string.h>
void concatenate(char str1[], char str2[]) {
strcat(str1, " "); // 在第一个字符串的末尾添加空格
strcat(str1, str2); // 将第二个字符串接在后面
}
int main() {
char str1[100], str2[100];
printf("请输入第一个字符串:");
scanf("%s", str1);
printf("请输入第二个字符串:");
scanf("%s", str2);
concatenate(str1, str2);
printf("合并后的字符串是:%s\n", str1);
return 0;
}
```
这个函数将两个字符串合并成一个字符串,并在它们之间添加一个空格。在主函数中,我们输入两个字符串,调用 `concatenate` 函数,然后输出合并后的字符串。希望这个示例可以帮助你。
用C语言求一个带空格的字符串的长度,长度包括空格,字符串末尾带空格
可以使用C语言的标准库函数`strlen()`来求字符串长度,但是`strlen()`函数会在遇到空格时停止计数,因此不能直接使用。
一种方法是使用字符数组和循环来遍历字符串,直到遇到字符串末尾的空字符`\0`,然后统计字符的个数即可。例如:
```c
#include <stdio.h>
int main() {
char str[100] = "hello world "; // 带空格的字符串
int len = 0; // 字符串长度
int i = 0; // 循环计数器
// 遍历字符串,直到遇到字符串末尾的空字符'\0'
while (str[i] != '\0') {
len++; // 统计字符的个数
i++; // 计数器加1
}
printf("字符串 %s 的长度为 %d\n", str, len);
return 0;
}
```
输出结果为:
```
字符串 hello world 的长度为 12
```
注意,上述方法计算的字符串长度包括空格和字符串末尾的空字符`\0`。
阅读全文
相关推荐
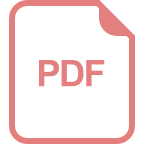
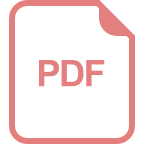
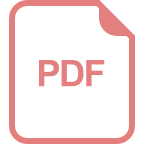












