在vue3中怎么写一段localStorage加持的本地历史记录,代码写详细点
时间: 2023-06-27 18:02:52 浏览: 141
在Vue3中,可以通过以下步骤来实现localStorage加持的本地历史记录:
1. 创建一个历史记录数组,用于存储用户的历史记录。
```
const history = [];
```
2. 在组件中监听输入框的输入事件,并将用户输入的内容添加到历史记录数组中。
```
<template>
<div>
<input type="text" v-model="inputValue" @keyup.enter="handleInputEnter" />
</div>
</template>
<script>
import { ref } from 'vue';
export default {
setup() {
const inputValue = ref('');
const handleInputEnter = () => {
history.push(inputValue.value);
localStorage.setItem('history', JSON.stringify(history));
inputValue.value = '';
};
return { inputValue, handleInputEnter };
}
};
</script>
```
3. 在组件初始化时,从localStorage中获取历史记录数组并赋值给history变量。
```
<script>
import { ref, onMounted } from 'vue';
export default {
setup() {
const history = ref([]);
onMounted(() => {
const historyFromStorage = localStorage.getItem('history');
if (historyFromStorage) {
history.value = JSON.parse(historyFromStorage);
}
});
return { history };
}
};
</script>
```
4. 渲染历史记录列表,并在点击每一项时将其赋值给输入框。
```
<template>
<div>
<input type="text" v-model="inputValue" @keyup.enter="handleInputEnter" />
<ul>
<li v-for="(item, index) in history" :key="index" @click="handleHistoryItemClick(item)">
{{ item }}
</li>
</ul>
</div>
</template>
<script>
import { ref, onMounted } from 'vue';
export default {
setup() {
const history = ref([]);
const inputValue = ref('');
onMounted(() => {
const historyFromStorage = localStorage.getItem('history');
if (historyFromStorage) {
history.value = JSON.parse(historyFromStorage);
}
});
const handleInputEnter = () => {
history.value.push(inputValue.value);
localStorage.setItem('history', JSON.stringify(history.value));
inputValue.value = '';
};
const handleHistoryItemClick = (item) => {
inputValue.value = item;
};
return { history, inputValue, handleInputEnter, handleHistoryItemClick };
}
};
</script>
```
以上代码实现了一个简单的localStorage加持的本地历史记录功能,可以根据需要进行更改和扩展。
阅读全文
相关推荐
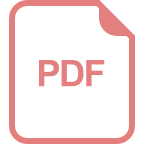
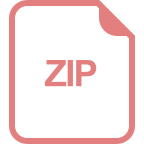
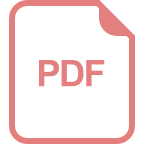
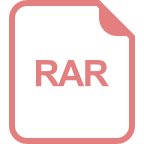
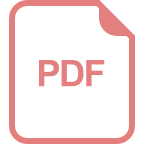
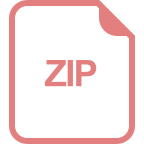
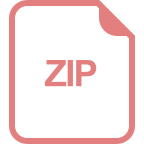
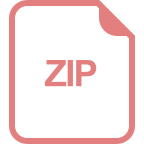
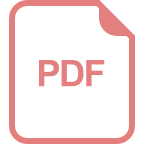
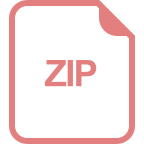
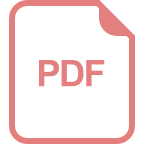
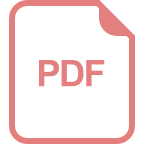
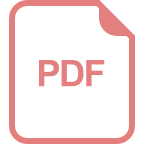
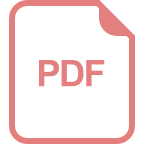
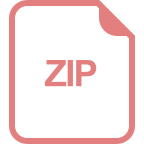
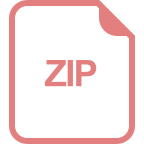
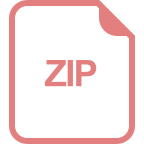
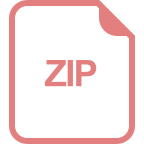