实验目标 掌握Java Swing图形用户界面编程 实验步骤 1.实现页面菜单,菜单项包括按名字读取、按学号读取、按班级读取; 2.实现搜索框,用于输入名字或学号,根据名字或学号查询学生信息; 3.实现班级下拉列表,用于选择班级并按班级查询学生信息。 4.实现学生信息展示功能,展示从数据库中查询到的学生信息;
时间: 2023-12-10 09:37:44 浏览: 138
好的,下面是一个简单的Java Swing图形用户界面编程示例,实现了上述四个功能:
1. 实现页面菜单:
```
JMenuBar menuBar = new JMenuBar();
JMenu fileMenu = new JMenu("文件");
JMenuItem nameItem = new JMenuItem("按名字读取");
JMenuItem idItem = new JMenuItem("按学号读取");
JMenuItem classItem = new JMenuItem("按班级读取");
fileMenu.add(nameItem);
fileMenu.add(idItem);
fileMenu.add(classItem);
menuBar.add(fileMenu);
```
2. 实现搜索框:
```
JTextField searchField = new JTextField(20);
JButton searchButton = new JButton("搜索");
searchButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String searchKey = searchField.getText();
// 根据名字或学号查询学生信息
// ...
}
});
```
3. 实现班级下拉列表:
```
String[] classList = {"一班", "二班", "三班"};
JComboBox<String> classBox = new JComboBox<String>(classList);
classBox.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String selectedClass = (String)classBox.getSelectedItem();
// 按班级查询学生信息
// ...
}
});
```
4. 实现学生信息展示功能:
```
JTable table = new JTable(data, columnNames);
JScrollPane scrollPane = new JScrollPane(table);
```
其中,`data`是从数据库中查询到的学生信息,`columnNames`是表格的列名。将`scrollPane`添加到窗口中即可展示学生信息。
完整代码示例:
```
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class StudentGUI extends JFrame {
public StudentGUI() {
// 实现页面菜单
JMenuBar menuBar = new JMenuBar();
JMenu fileMenu = new JMenu("文件");
JMenuItem nameItem = new JMenuItem("按名字读取");
JMenuItem idItem = new JMenuItem("按学号读取");
JMenuItem classItem = new JMenuItem("按班级读取");
fileMenu.add(nameItem);
fileMenu.add(idItem);
fileMenu.add(classItem);
menuBar.add(fileMenu);
setJMenuBar(menuBar);
// 实现搜索框
JPanel searchPanel = new JPanel();
JLabel searchLabel = new JLabel("搜索:");
JTextField searchField = new JTextField(20);
JButton searchButton = new JButton("搜索");
searchButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String searchKey = searchField.getText();
// 根据名字或学号查询学生信息
// ...
}
});
searchPanel.add(searchLabel);
searchPanel.add(searchField);
searchPanel.add(searchButton);
getContentPane().add(searchPanel, BorderLayout.NORTH);
// 实现班级下拉列表
JPanel classPanel = new JPanel();
JLabel classLabel = new JLabel("班级:");
String[] classList = {"一班", "二班", "三班"};
JComboBox<String> classBox = new JComboBox<String>(classList);
classBox.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String selectedClass = (String)classBox.getSelectedItem();
// 按班级查询学生信息
// ...
}
});
classPanel.add(classLabel);
classPanel.add(classBox);
getContentPane().add(classPanel, BorderLayout.WEST);
// 实现学生信息展示功能
String[] columnNames = {"学号", "姓名", "班级"};
Object[][] data = {{"001", "张三", "一班"},
{"002", "李四", "二班"},
{"003", "王五", "三班"}};
JTable table = new JTable(data, columnNames);
JScrollPane scrollPane = new JScrollPane(table);
getContentPane().add(scrollPane, BorderLayout.CENTER);
setTitle("学生信息管理");
setSize(500, 300);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
StudentGUI gui = new StudentGUI();
gui.setVisible(true);
}
}
```
阅读全文
相关推荐
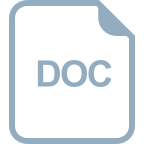






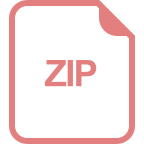
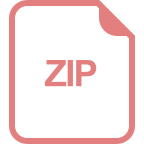
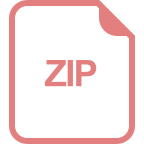
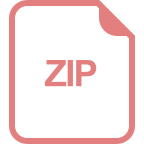





