springboot redis哨兵
时间: 2023-08-28 18:06:34 浏览: 112
Spring Boot中使用Redis哨兵模式可以提高Redis的高可用性和可靠性。下面是使用Spring Boot集成Redis哨兵模式的基本步骤:
1. 首先,在`pom.xml`文件中添加Redis和Spring Data Redis的依赖:
```xml
<dependencies>
<!-- Redis -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
</dependencies>
```
2. 在`application.properties`(或`application.yml`)文件中配置Redis哨兵模式的相关参数:
```properties
spring.redis.sentinel.master=your-master-name
spring.redis.sentinel.nodes=host1:port1,host2:port2,host3:port3
```
其中,`your-master-name`是你的Redis主节点的名称,`host1:port1,host2:port2,host3:port3`是你的Redis哨兵节点的主机和端口列表。
3. 创建一个Redis配置类,用于配置Redis连接工厂和Redis模板:
```java
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.connection.RedisSentinelConfiguration;
import org.springframework.data.redis.connection.jedis.JedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.StringRedisSerializer;
@Configuration
public class RedisConfig {
// 配置Redis连接工厂
public RedisConnectionFactory redisConnectionFactory() {
RedisSentinelConfiguration sentinelConfig = new RedisSentinelConfiguration()
.master("your-master-name")
.sentinel("host1", port1)
.sentinel("host2", port2)
.sentinel("host3", port3);
return new JedisConnectionFactory(sentinelConfig);
}
// 配置Redis模板
public RedisTemplate<String, String> redisTemplate() {
RedisTemplate<String, String> redisTemplate = new RedisTemplate<>();
redisTemplate.setConnectionFactory(redisConnectionFactory());
redisTemplate.setDefaultSerializer(new StringRedisSerializer());
return redisTemplate;
}
}
```
在以上示例中,需要根据你的实际配置修改`your-master-name`、`host1`、`port1`等参数。
4. 在需要使用Redis的地方注入`RedisTemplate`并使用它来操作Redis数据:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.stereotype.Service;
@Service
public class MyService {
@Autowired
private RedisTemplate<String, String> redisTemplate;
public void saveData(String key, String value) {
redisTemplate.opsForValue().set(key, value);
}
public String getData(String key) {
return redisTemplate.opsForValue().get(key);
}
}
```
在上述示例中,我们使用`redisTemplate`来执行一些常见的Redis操作,比如设置值和获取值。
这样,就完成了Spring Boot中使用Redis哨兵模式的集成配置和使用。你可以根据自己的需求进一步扩展和优化。
阅读全文
相关推荐
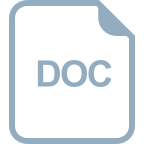
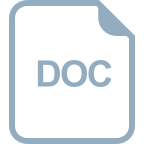
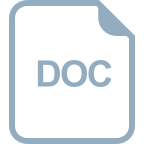






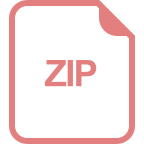
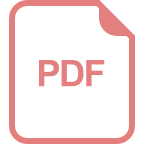
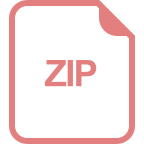
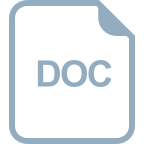





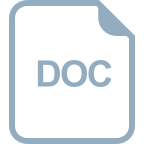