Python 定时抓取onedrive文件到本地文件夹
时间: 2024-05-27 13:07:12 浏览: 21
可以使用Python中的schedule模块和onedrive模块来实现定时抓取onedrive文件到本地文件夹。
首先需要安装schedule和onedrive模块,可以使用以下命令进行安装:
```
pip install schedule
pip install onedrive-sdk-python
```
接下来,你需要获得onedrive的API凭证,具体步骤可以参考Microsoft官方文档:https://docs.microsoft.com/en-us/onedrive/developer/rest-api/getting-started/app-registration?view=odsp-graph-online
获取到API凭证后,你可以使用以下代码实现定时抓取onedrive文件到本地文件夹:
```python
import schedule
import time
import os
from onedrivesdk import (
OneDriveClient,
http,
AuthProvider
)
# 定义抓取间隔时间(秒)
INTERVAL = 60 * 10
# 定义本地文件夹路径和要抓取的onedrive文件路径
LOCAL_FOLDER_PATH = "D:/onedrive_files"
ONEDRIVE_FILE_PATH = "/Documents/file.txt"
# 定义onedrive API凭证信息
CLIENT_ID = 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
CLIENT_SECRET = 'xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'
REDIRECT_URI = 'http://localhost:8080'
REFRESH_TOKEN = 'xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'
# 初始化auth_provider和client
auth_provider = AuthProvider(
client_id=CLIENT_ID,
client_secret=CLIENT_SECRET,
redirect_uri=REDIRECT_URI,
refresh_token=REFRESH_TOKEN
)
client = OneDriveClient(auth_provider=auth_provider)
def download_file():
# 获取onedrive文件的下载链接
item = client.item(path=ONEDRIVE_FILE_PATH)
download_url = client.item(drive='me', id=item.id).get('@microsoft.graph.downloadUrl')
# 下载文件到本地文件夹
response = http.get(download_url)
file_name = os.path.basename(ONEDRIVE_FILE_PATH)
with open(os.path.join(LOCAL_FOLDER_PATH, file_name), "wb") as f:
f.write(response.content)
print("File downloaded successfully!")
schedule.every(INTERVAL).seconds.do(download_file)
while True:
schedule.run_pending()
time.sleep(1)
```
以上代码定义了定时抓取间隔时间、本地文件夹路径、要抓取的onedrive文件路径以及API凭证信息。在`download_file`函数中,首先使用`client.item`方法获取onedrive文件的信息,然后使用`client.item(drive='me', id=item.id).get('@microsoft.graph.downloadUrl')`方法获取文件的下载链接,最后使用`http.get`方法下载文件到本地文件夹。
如果你想要抓取其他类型的文件,只需要将`ONEDRIVE_FILE_PATH`更改为相应的路径即可。
相关推荐
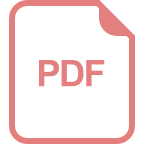














