java实现将rtsp流转换为websocket-flv推送前端播放的代码
时间: 2023-06-18 10:04:12 浏览: 150
实现将RTSP流转换为WebSocket-FLV推送前端播放的代码,可以使用开源的Java框架Netty进行实现。下面是一个简单的示例代码:
```java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.Channel;
import io.netty.channel.ChannelFutureListener;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelPipeline;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
import io.netty.handler.codec.http.HttpContentCompressor;
import io.netty.handler.codec.http.HttpObjectAggregator;
import io.netty.handler.codec.http.HttpServerCodec;
import io.netty.handler.codec.rtsp.RtspDecoder;
import io.netty.handler.codec.rtsp.RtspEncoder;
import io.netty.handler.stream.ChunkedWriteHandler;
import io.netty.handler.codec.http.websocketx.WebSocketServerProtocolHandler;
import io.netty.handler.stream.ChunkedWriteHandler;
public class RtspToWebSocketServer {
private final int port;
public RtspToWebSocketServer(int port) {
this.port = port;
}
public void run() throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup(1);
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
// 添加RTSP协议编码解码器
pipeline.addLast("rtspEncoder", new RtspEncoder());
pipeline.addLast("rtspDecoder", new RtspDecoder());
// 添加HTTP协议编码解码器
pipeline.addLast("httpServerCodec", new HttpServerCodec());
pipeline.addLast("httpObjectAggregator", new HttpObjectAggregator(65536));
pipeline.addLast("httpContentCompressor", new HttpContentCompressor());
// 添加WebSocket协议处理器
pipeline.addLast("webSocketHandler", new WebSocketServerProtocolHandler("/ws"));
pipeline.addLast("chunkedWriteHandler", new ChunkedWriteHandler());
// 添加自定义的RTSP转WebSocket处理器
pipeline.addLast("rtspToWebSocketHandler", new RtspToWebSocketHandler());
}
});
Channel ch = b.bind(port).sync().channel();
ch.closeFuture().addListener((ChannelFutureListener) future -> {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
});
System.out.println("RTSP to WebSocket server started at port " + port + '.');
System.out.println("Open your browser and navigate to http://127.0.0.1:" + port + '/');
ch.closeFuture().sync();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
int port = 8080;
new RtspToWebSocketServer(port).run();
}
}
```
上述代码中,我们使用了Netty框架来实现RTSP转WebSocket的功能。具体步骤如下:
1. 创建一个`ServerBootstrap`对象,用于启动服务器;
2. 添加`RtspEncoder`和`RtspDecoder`,用于编解码RTSP协议;
3. 添加`HttpServerCodec`、`HttpObjectAggregator`和`HttpContentCompressor`,用于编解码HTTP协议;
4. 添加`WebSocketServerProtocolHandler`,用于处理WebSocket协议;
5. 添加`ChunkedWriteHandler`,用于处理大数据流;
6. 添加自定义的`RtspToWebSocketHandler`,用于将RTSP流转换为WebSocket-FLV流;
7. 绑定端口并启动服务器。
需要注意的是,上述代码只是一个简单的示例,实际应用场景中可能需要根据具体需求进行修改。
相关推荐
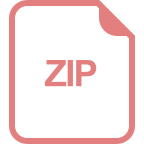
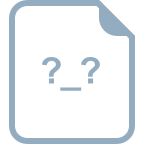














