编写程序,从键盘输入 n (n<10)本书的名称和定价并存入结构数组中,查找并输出其中定价最高和最低的书的名称和定价。 输出格式语句: printf("highest price: %.1f, %s\n", ); printf("lowest price: %.1f, %s\n",); 输入输出示例:括号内为说明,无需输入输出 输入样例: 3 (n=3) Programming in C 21.5 Programming in VB 18.5 Programming in Delphi 25 输出样例: highest price: 25.0, Programming in Delphi lowest price: 18.5, Programming in VB
时间: 2023-11-27 21:54:06 浏览: 192
以下是一个可能的解决方案:
```c
#include <stdio.h>
#include <string.h>
#define MAX_BOOKS 10
struct Book {
char name[50];
float price;
};
int main() {
int n;
struct Book books[MAX_BOOKS];
float highest_price = 0, lowest_price = 100000; // 初始化定价范围
printf("Enter the number of books (n<10): ");
scanf("%d", &n);
// 读入书籍信息
for (int i = 0; i < n; i++) {
printf("Enter the name and price of book %d: ", i+1);
scanf("%s%f", books[i].name, &books[i].price);
// 更新最高和最低价格
if (books[i].price > highest_price) {
highest_price = books[i].price;
}
if (books[i].price < lowest_price) {
lowest_price = books[i].price;
}
}
// 输出结果
printf("highest price: %.1f, %s\n", highest_price, books[0].name); // 初始化为第一本书籍
printf("lowest price: %.1f, %s\n", lowest_price, books[0].name); // 初始化为第一本书籍
for (int i = 1; i < n; i++) {
if (books[i].price == highest_price) {
printf("highest price: %.1f, %s\n", highest_price, books[i].name);
}
if (books[i].price == lowest_price) {
printf("lowest price: %.1f, %s\n", lowest_price, books[i].name);
}
}
return 0;
}
```
该程序首先从键盘读入书籍数量 `n`,然后按顺序读入每一本书的名称和价格,并在读入时更新最高和最低价格。最后,输出包含最高和最低价格的书籍名称和价格。注意,如果有多本书价格相同,则它们都会被输出。
阅读全文
相关推荐
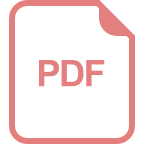
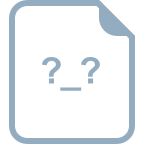
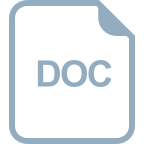










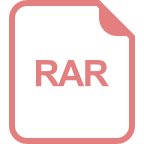
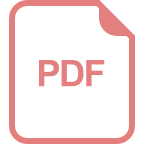
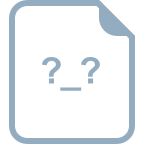
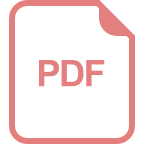