用C语言写一个 二叉树遍历算法
时间: 2023-03-15 22:19:10 浏览: 62
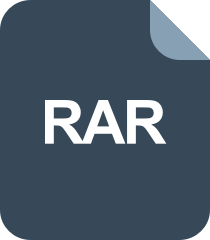
C语言实现二叉树遍历

C语言中二叉树遍历算法有三种:先序遍历、中序遍历和后序遍历。
先序遍历的代码如下:
```c
void preorder(struct node* root) {
if (root != NULL) {
printf("%d ", root->data);
preorder(root->left);
preorder(root->right);
}
}
```
中序遍历的代码如下:
```c
void inorder(struct node* root) {
if (root != NULL) {
inorder(root->left);
printf("%d ", root->data);
inorder(root->right);
}
}
```
后序遍历的代码如下:
```c
void postorder(struct node* root) {
if (root != NULL) {
postorder(root->left);
postorder(root->right);
printf("%d ", root->data);
}
}
```
注意上面的代码假设二叉树的结构体定义如下:
```c
struct node {
int data;
struct node* left;
struct node* right;
};
```
使用时,可以如下调用:
```c
preorder(root);
inorder(root);
postorder(root);
```
请注意,这些代码只是示例,实际应用中可能需要根据需求进行修改。
阅读全文
相关推荐


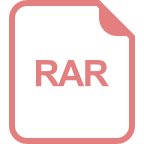
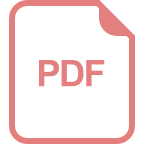
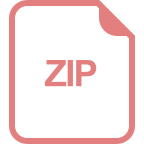