粒子群算法求解多目标规划c语言
时间: 2023-08-24 08:40:07 浏览: 133
粒子群算法(Particle Swarm Optimization,简称PSO)是一种基于群体智能的优化算法,常用于求解多目标规划问题。下面是一个使用C语言实现PSO求解多目标规划的简单示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define POP_SIZE 50 // 种群大小
#define DIM 2 // 变量维度
#define OBJ_NUM 2 // 目标函数个数
#define MAX_ITER 1000 // 最大迭代次数
/* 目标函数 */
void obj_func(double x[], double f[])
{
f[0] = pow(x[0], 2) + pow(x[1], 2);
f[1] = pow(x[0] - 1, 2) + pow(x[1] - 1, 2);
}
/* 初始化粒子群 */
void init_swarm(double swarm[][DIM], double v[][DIM], double pbest[][DIM], double gbest[])
{
int i, j;
for (i = 0; i < POP_SIZE; i++) {
for (j = 0; j < DIM; j++) {
swarm[i][j] = (double)rand() / RAND_MAX; // 随机初始化位置
v[i][j] = 0; // 初始速度为0
pbest[i][j] = swarm[i][j]; // 初始个体最优为初始位置
}
obj_func(swarm[i], &pbest[i][DIM]); // 计算个体最优解
if (i == 0 || pbest[i][DIM] < gbest[DIM]) {
for (j = 0; j < DIM; j++) {
gbest[j] = pbest[i][j]; // 初始全局最优为第一个个体的位置
}
gbest[DIM] = pbest[i][DIM];
}
}
}
/* 更新速度和位置 */
void update_swarm(double swarm[][DIM], double v[][DIM], double pbest[][DIM], double gbest[])
{
int i, j;
double w = 0.7; // 惯性权重
double c1 = 1.5; // 学习因子
double c2 = 1.5;
for (i = 0; i < POP_SIZE; i++) {
for (j = 0; j < DIM; j++) {
v[i][j] = w * v[i][j] + c1 * (pbest[i][j] - swarm[i][j]) * (double)rand() / RAND_MAX + c2 * (gbest[j] - swarm[i][j]) * (double)rand() / RAND_MAX; // 更新速度
swarm[i][j] += v[i][j]; // 更新位置
if (swarm[i][j] < 0) {
swarm[i][j] = 0;
}
if (swarm[i][j] > 1) {
swarm[i][j] = 1;
}
}
obj_func(swarm[i], &pbest[i][DIM]); // 计算个体最优解
if (pbest[i][DIM] < gbest[DIM]) {
for (j = 0; j < DIM; j++) {
gbest[j] = pbest[i][j]; // 更新全局最优
}
gbest[DIM] = pbest[i][DIM];
}
}
}
/* 主函数 */
int main()
{
double swarm[POP_SIZE][DIM]; // 粒子群当前位置
double v[POP_SIZE][DIM]; // 粒子群当前速度
double pbest[POP_SIZE][DIM + OBJ_NUM]; // 粒子群当前个体最优
double gbest[DIM + OBJ_NUM]; // 粒子群当前全局最优
int iter = 0; // 当前迭代次数
init_swarm(swarm, v, pbest, gbest); // 初始化粒子群
while (iter < MAX_ITER) {
update_swarm(swarm, v, pbest, gbest); // 更新粒子群
iter++;
}
printf("The optimal solution is: (%lf, %lf), with the objective values: (%lf, %lf)\n", gbest[0], gbest[1], gbest[2], gbest[3]); // 输出最优解
return 0;
}
```
在上述代码中,`init_swarm`函数用于初始化粒子群,`update_swarm`函数用于更新粒子群,`obj_func`函数为目标函数,其输入为当前位置`x`,输出为目标函数值`f`。在每次迭代中,粒子群的速度和位置根据公式进行更新,直到达到最大迭代次数为止。最终输出全局最优解和对应的目标函数值。
阅读全文
相关推荐
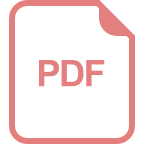
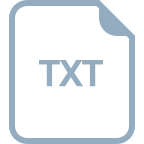
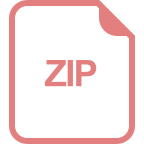
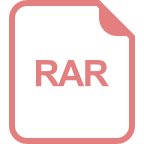
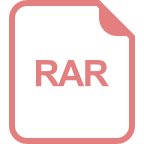
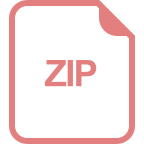
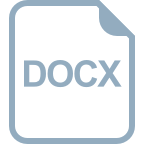
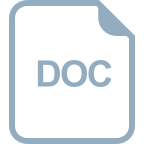
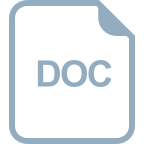
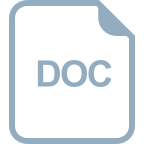
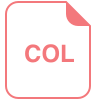
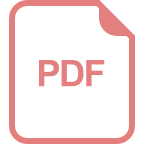
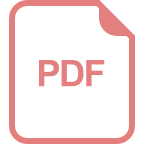