4_jfoenix_Scene Builder,JFXComboBox,切换场景
时间: 2023-06-26 07:06:42 浏览: 125
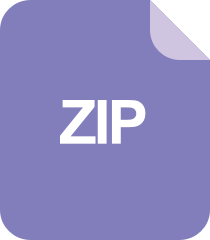
菜单 场景切换

好的,首先我们需要使用JavaFX的Scene Builder工具来创建GUI界面。您可以下载并安装Scene Builder,然后使用它来创建您需要的用户界面。
在创建界面时,您可以使用JFXComboBox控件来实现下拉菜单,该控件可以让用户从预定义的选项中进行选择。
接下来,您可以使用JavaFX的Scene类来创建不同的场景,并使用JFXComboBox控件中的事件处理程序来切换场景。例如,您可以在下拉菜单中添加选项来切换到不同的场景。
以下是一个简单的示例代码,演示了如何在JavaFX中使用JFXComboBox控件和Scene类来切换场景:
```java
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.ComboBox;
import javafx.scene.layout.StackPane;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class SceneSwitching extends Application {
private Stage stage;
private Scene scene1, scene2, scene3;
@Override
public void start(Stage primaryStage) throws Exception {
stage = primaryStage;
// Create the first scene
Label label1 = new Label("Scene 1");
StackPane layout1 = new StackPane(label1);
scene1 = new Scene(layout1, 300, 200);
// Create the second scene
Label label2 = new Label("Scene 2");
StackPane layout2 = new StackPane(label2);
scene2 = new Scene(layout2, 300, 200);
// Create the third scene
Label label3 = new Label("Scene 3");
StackPane layout3 = new StackPane(label3);
scene3 = new Scene(layout3, 300, 200);
// Create the ComboBox control
ObservableList<String> options = FXCollections.observableArrayList("Scene 1", "Scene 2", "Scene 3");
ComboBox<String> comboBox = new ComboBox<>(options);
comboBox.setOnAction(e -> switchScene(comboBox.getValue()));
// Create the layout for the main scene
VBox mainLayout = new VBox(comboBox);
mainLayout.setPadding(new Insets(10));
mainLayout.setSpacing(10);
Scene mainScene = new Scene(mainLayout, 300, 200);
stage.setScene(mainScene);
stage.show();
}
private void switchScene(String sceneName) {
switch (sceneName) {
case "Scene 1":
stage.setScene(scene1);
break;
case "Scene 2":
stage.setScene(scene2);
break;
case "Scene 3":
stage.setScene(scene3);
break;
default:
break;
}
}
public static void main(String[] args) {
launch(args);
}
}
```
在此示例中,我们创建了三个不同的场景,并使用ComboBox控件添加了选项,以便用户可以从中进行选择。当用户选择选项时,我们使用switch语句来切换到相应的场景。注意,我们在ComboBox控件的事件处理程序中调用了switchScene方法来切换场景。
您可以根据自己的需求,使用类似的代码来实现场景切换功能。
阅读全文
相关推荐
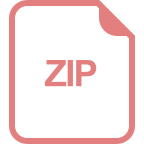
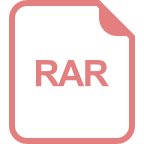
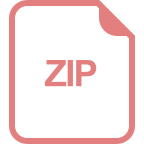
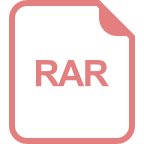
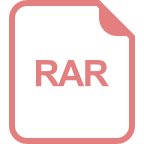
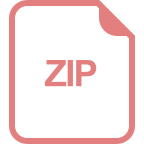
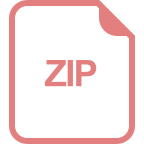
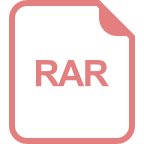
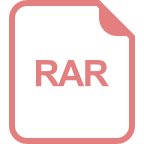
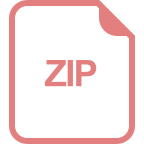
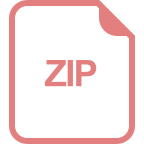
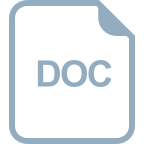