Java如何给链表地址赋值
时间: 2024-09-10 08:16:50 浏览: 45
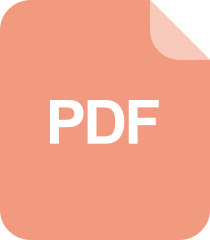
Java语言实现反转链表代码示例
在Java中,链表通常是通过使用`LinkedList`类或者自定义链表结构来实现的。`LinkedList`类是Java集合框架的一部分,提供了添加、删除和访问链表节点的简便方法。如果要给链表地址赋值,通常是将一个节点或者一组节点插入到链表中的特定位置,或者将一个链表的内容复制到另一个链表中。
以下是一个基本示例,说明如何在`LinkedList`中添加元素:
```java
import java.util.LinkedList;
public class LinkedListExample {
public static void main(String[] args) {
// 创建一个LinkedList实例
LinkedList<String> linkedList = new LinkedList<>();
// 给链表赋值,添加元素
linkedList.add("元素1");
linkedList.add("元素2");
linkedList.add("元素3");
// 打印链表中的所有元素
System.out.println(linkedList);
}
}
```
如果要通过自定义链表节点的方式手动管理链表的地址赋值,可以定义一个节点类和一个链表类:
```java
class ListNode {
int val;
ListNode next;
ListNode(int x) {
val = x;
next = null;
}
}
class LinkedListManual {
ListNode head;
// 添加元素到链表末尾
public void add(int value) {
ListNode newNode = new ListNode(value);
if (head == null) {
head = newNode;
} else {
ListNode current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
}
// 打印链表
public void printList() {
ListNode current = head;
while (current != null) {
System.out.print(current.val + " -> ");
current = current.next;
}
System.out.println("null");
}
}
// 使用自定义链表
public class LinkedListManualExample {
public static void main(String[] args) {
LinkedListManual list = new LinkedListManual();
list.add(1);
list.add(2);
list.add(3);
list.printList();
}
}
```
在上述例子中,`LinkedListManual`类通过`add`方法自行维护链表的节点连接,即地址赋值。
阅读全文
相关推荐


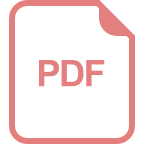
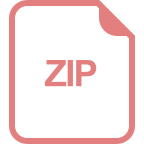
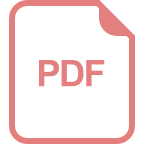
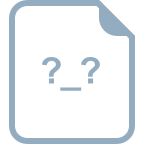
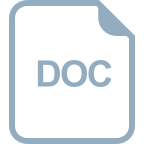
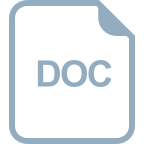
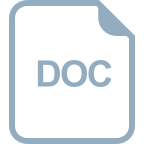
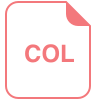
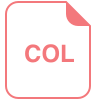





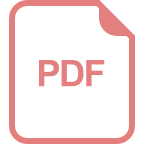