Java对目录下的zip进行解压
时间: 2023-07-01 09:22:13 浏览: 117
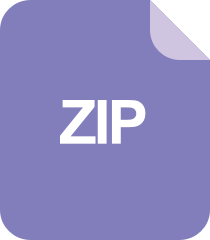
JAVA实现目录及文件的zip压缩和解压
可以使用Java中的ZipInputStream类对zip文件进行解压缩,示例代码如下:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
public class Unzip {
public static void main(String[] args) {
String zipFilePath = "path/to/zip/file.zip";
String destDirectory = "path/to/destination/directory";
byte[] buffer = new byte[1024];
try {
// create output directory if it doesn't exist
File folder = new File(destDirectory);
if (!folder.exists()) {
folder.mkdir();
}
// get the zip file content
ZipInputStream zis = new ZipInputStream(new FileInputStream(zipFilePath));
// get the zipped file list entry
ZipEntry ze = zis.getNextEntry();
while (ze != null) {
String fileName = ze.getName();
File newFile = new File(destDirectory + File.separator + fileName);
// create all non exists folders
new File(newFile.getParent()).mkdirs();
FileOutputStream fos = new FileOutputStream(newFile);
int len;
while ((len = zis.read(buffer)) > 0) {
fos.write(buffer, 0, len);
}
fos.close();
ze = zis.getNextEntry();
}
zis.closeEntry();
zis.close();
System.out.println("File unzipped successfully");
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
```
其中,`zipFilePath`表示zip文件的路径,`destDirectory`表示解压缩后文件存放的目录。程序首先创建了存放解压缩文件的目录,然后使用`ZipInputStream`类读取zip文件中的文件列表,并逐一解压缩到指定目录中。
阅读全文
相关推荐
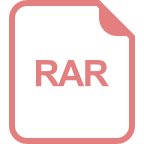
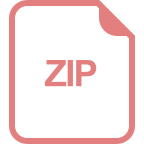
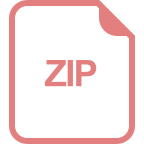
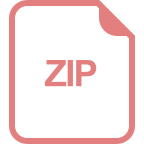
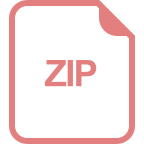
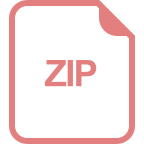
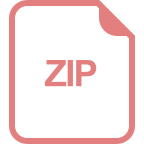
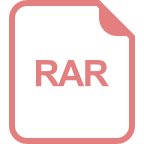
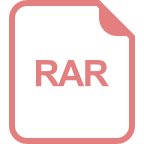
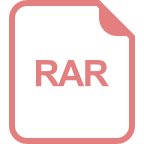
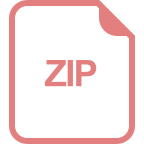
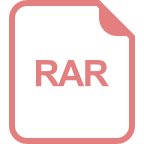
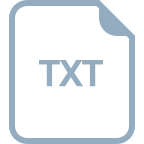