spooling技术例程库
时间: 2023-12-17 19:01:19 浏览: 70
spooling技术是一种用于优化计算机系统资源利用的技术,用来解决多个用户同时访问共享资源时的冲突和效率问题。而例程库则是一组提供给程序员使用的预定义例程或函数的集合。
在使用spooling技术时,系统会将用户的任务临时存储到磁盘或内存中,然后逐个处理,从而实现并发处理多个任务的目的。这样一来,多个用户就可以同时提交任务而不会产生冲突,也不需要等待其他任务的完成。
例程库是一个由各种常用函数和程序组成的库,可以为程序员提供快速、高效的开发工具。这些例程库中包含了各种常用的功能函数,例如输入输出操作、字符串处理、数学运算等。程序员可以通过调用这些例程来完成特定的任务,而不需要从头开始编写整个程序,大大提高了开发效率和代码质量。
结合spooling技术和例程库的优势,可以实现更高效的任务处理。多个用户可以同时提交任务,并且可以利用例程库中提供的函数来完成特定的任务,减少了代码开发的重复劳动。同时,通过spooling技术,可以更加充分地利用计算机系统的资源,提高系统的性能和效率。
总之,spooling技术和例程库的结合可以为计算机系统的任务处理提供更高效、更快速的解决方案,提高了系统的并发性和资源利用率。这对于用户和程序员来说都是非常有益的。
相关问题
实现 I/O设备管理spooling技术例程库的c代码
以下是一个简单的 I/O 设备管理 spooling 技术例程库的 C 代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <pthread.h>
#define MAX_QUEUE_SIZE 10
#define MAX_LINE_LENGTH 1024
typedef struct {
char *line;
int length;
} Line;
typedef struct {
Line queue[MAX_QUEUE_SIZE];
int front;
int rear;
int size;
pthread_mutex_t mutex;
pthread_cond_t cond_full;
pthread_cond_t cond_empty;
} Spooler;
void init_spooler(Spooler *spooler) {
spooler->front = 0;
spooler->rear = 0;
spooler->size = 0;
pthread_mutex_init(&spooler->mutex, NULL);
pthread_cond_init(&spooler->cond_full, NULL);
pthread_cond_init(&spooler->cond_empty, NULL);
}
void destroy_spooler(Spooler *spooler) {
pthread_mutex_destroy(&spooler->mutex);
pthread_cond_destroy(&spooler->cond_full);
pthread_cond_destroy(&spooler->cond_empty);
}
void enqueue(Spooler *spooler, const char *line) {
pthread_mutex_lock(&spooler->mutex);
while (spooler->size == MAX_QUEUE_SIZE) {
pthread_cond_wait(&spooler->cond_full, &spooler->mutex);
}
spooler->queue[spooler->rear].length = strlen(line);
spooler->queue[spooler->rear].line = malloc(spooler->queue[spooler->rear].length + 1);
strcpy(spooler->queue[spooler->rear].line, line);
spooler->rear = (spooler->rear + 1) % MAX_QUEUE_SIZE;
spooler->size++;
pthread_cond_signal(&spooler->cond_empty);
pthread_mutex_unlock(&spooler->mutex);
}
Line dequeue(Spooler *spooler) {
pthread_mutex_lock(&spooler->mutex);
while (spooler->size == 0) {
pthread_cond_wait(&spooler->cond_empty, &spooler->mutex);
}
Line line = spooler->queue[spooler->front];
spooler->front = (spooler->front + 1) % MAX_QUEUE_SIZE;
spooler->size--;
pthread_cond_signal(&spooler->cond_full);
pthread_mutex_unlock(&spooler->mutex);
return line;
}
void *spooler_thread(void *arg) {
Spooler *spooler = (Spooler *) arg;
while (1) {
Line line = dequeue(spooler);
printf("Printing: %s\n", line.line);
free(line.line);
}
}
void print(const char *line, Spooler *spooler) {
enqueue(spooler, line);
}
int main() {
Spooler spooler;
init_spooler(&spooler);
pthread_t thread;
pthread_create(&thread, NULL, spooler_thread, &spooler);
char line[MAX_LINE_LENGTH];
while (fgets(line, MAX_LINE_LENGTH, stdin)) {
print(line, &spooler);
}
destroy_spooler(&spooler);
pthread_join(thread, NULL);
return 0;
}
```
这段代码定义了一个 Spooler 结构体,其中包含一个循环队列,一个互斥锁和两个条件变量。enqueue 函数将一行字符插入到队列中,dequeue 函数从队列中取出一行字符。spooler_thread 函数是一个长期运行的线程,它不断从队列中取出字符并打印。
在 main 函数中,我们首先初始化一个 Spooler 实例,然后创建一个线程来处理队列中的字符。接下来,我们从标准输入读取字符,并将其插入到队列中。最后,我们销毁 Spooler 实例并等待线程结束。
操作系统课设假脱机技术spooling技术
好的,针对您的问题,我可以为您提供一些关于操作系统课设中的假脱机技术spooling技术的介绍。
Spooling技术是指在计算机系统中,为了使I/O设备与计算机的CPU之间达到同步工作,避免设备与CPU之间的等待,设立的一个I/O缓冲区。当一个进程请求I/O操作时,操作系统将其I/O请求放入缓冲区中等待处理,同时进程也可以继续运行。等到I/O设备空闲时,再从缓冲区中取出请求进行处理。
在操作系统课设中,Spooling技术通常用来模拟脱机打印功能。当用户需要打印文件时,文件会被放置到一个输出队列中,然后打印机会从输出队列中读取文件进行打印。这种技术可以让用户无需等待打印机完成打印,而是可以继续进行其他操作。
需要注意的是,Spooling技术可以提高计算机系统的性能,但也会占用系统的一定资源。因此,在实际应用中需要根据具体情况进行权衡和调整。
希望这些信息能对您有所帮助!
相关推荐
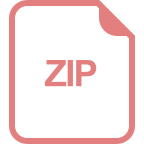
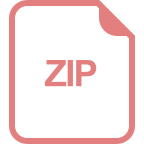
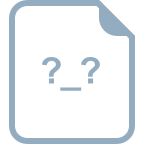













