Java有没有3D的凸壳算法
时间: 2023-12-01 10:32:01 浏览: 116
是的,Java有3D凸壳算法的实现。常用的算法包括QuickHull和JConvexHull。
QuickHull算法是一种基于分治法的凸壳算法,它的时间复杂度为O(nlogn)。JConvexHull是一个Java库,实现了几种凸壳算法,包括QuickHull、Graham-Scan、Chan和Kong等算法,它的使用非常方便。
以下是使用JConvexHull库进行3D凸壳计算的示例代码:
```java
import java.util.Arrays;
import java.util.List;
import org.jzy3d.chart.Chart;
import org.jzy3d.chart.controllers.mouse.camera.AWTMouseCameraController;
import org.jzy3d.chart.factories.AWTChartComponentFactory;
import org.jzy3d.chart.factories.ChartComponentFactory;
import org.jzy3d.colors.Color;
import org.jzy3d.maths.Coord3d;
import org.jzy3d.plot3d.primitives.Point;
import org.jzy3d.plot3d.primitives.Polygon;
import org.jzy3d.plot3d.primitives.Shape;
import org.jzy3d.plot3d.rendering.canvas.Quality;
import org.jzy3d.plot3d.rendering.view.modes.ViewBoundMode;
import com.vividsolutions.jts.geom.Coordinate;
import com.vividsolutions.jts.geom.Geometry;
import com.vividsolutions.jts.geom.GeometryFactory;
import com.vividsolutions.jts.geom.Polygon;
import org.apache.commons.collections.CollectionUtils;
import org.apache.commons.lang3.ArrayUtils;
import org.apache.commons.math3.geometry.euclidean.threed.Vector3D;
import org.apache.commons.math3.util.FastMath;
public class JConvexHullExample {
public static void main(String[] args) {
// Create a list of 3D points
List<Coord3d> points = Arrays.asList(
new Coord3d(-1, -1, -1),
new Coord3d(-1, -1, 1),
new Coord3d(-1, 1, -1),
new Coord3d(-1, 1, 1),
new Coord3d(1, -1, -1),
new Coord3d(1, -1, 1),
new Coord3d(1, 1, -1),
new Coord3d(1, 1, 1)
);
// Convert the list of 3D points to an array of JTS coordinates
Coordinate[] coordinates = new Coordinate[points.size()];
for (int i = 0; i < points.size(); i++) {
Coord3d p = points.get(i);
coordinates[i] = new Coordinate(p.x, p.y, p.z);
}
// Create a JTS geometry factory
GeometryFactory factory = new GeometryFactory();
// Create a JTS geometry from the array of coordinates
Geometry geometry = factory.createMultiPoint(coordinates);
// Compute the 3D convex hull using the QuickHull algorithm
org.jzy3d.plot3d.primitives.Polygon[] polygons = org.jzy3d.plot3d.primitives.Polygon
.toArray(JConvexHull.getConvexHull(geometry, false));
// Create a Jzy3d shape from the 3D convex hull
Shape shape = new Shape();
for (org.jzy3d.plot3d.primitives.Polygon polygon : polygons) {
Color color = new Color(FastMath.random(), FastMath.random(), FastMath.random());
Polygon p = new Polygon();
for (Point point : polygon.getPoints()) {
p.add(new Point(new Coord3d(point.x, point.y, point.z), color));
}
shape.add(p);
}
// Create a Jzy3d chart with the convex hull shape
Chart chart = AWTChartComponentFactory.chart(Quality.Advanced, ChartComponentFactory.Toolkit.offscreen());
chart.getScene().add(shape);
chart.getView().setBoundMode(ViewBoundMode.AUTO_FIT);
chart.getView().setCameraMouseController(new AWTMouseCameraController());
// Display the chart
chart.open("3D Convex Hull Example");
}
}
```
在这个示例中,我们使用JConvexHull库计算了一个由8个点组成的立方体的3D凸壳,并将结果可视化。
阅读全文
相关推荐
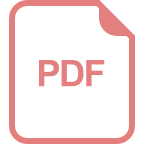
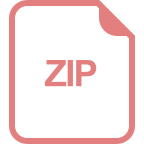
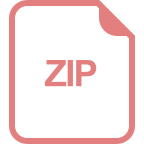
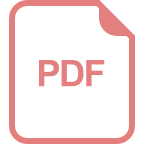
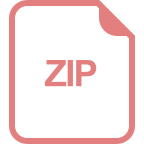
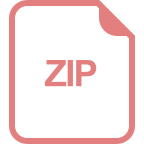
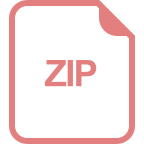
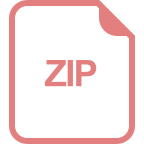
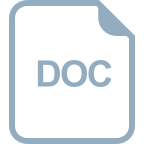
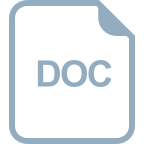
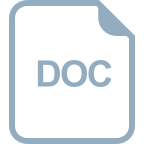
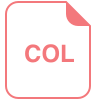
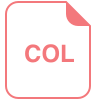
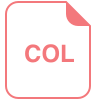
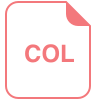
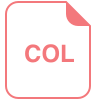