STM32F103读取BME280
时间: 2024-04-30 18:03:54 浏览: 10
BME280是一款数字式温湿度气压传感器,可以通过I2C或SPI接口与STM32F103进行通信。以下是使用I2C接口读取BME280的示例代码:
```c
#include "stm32f10x.h"
#include "i2c.h"
#define BME280_ADDRESS 0xEE
uint8_t bme280_read_byte(uint8_t reg)
{
uint8_t data;
I2C_Start();
I2C_SendByte(BME280_ADDRESS << 1);
I2C_WaitAck();
I2C_SendByte(reg);
I2C_WaitAck();
I2C_Start();
I2C_SendByte((BME280_ADDRESS << 1) | 0x01);
I2C_WaitAck();
data = I2C_ReceiveByte();
I2C_Stop();
return data;
}
int16_t bme280_read_short(uint8_t reg)
{
uint8_t data[2];
int16_t value;
I2C_Start();
I2C_SendByte(BME280_ADDRESS << 1);
I2C_WaitAck();
I2C_SendByte(reg);
I2C_WaitAck();
I2C_Start();
I2C_SendByte((BME280_ADDRESS << 1) | 0x01);
I2C_WaitAck();
data[0] = I2C_ReceiveByte();
I2C_Ack();
data[1] = I2C_ReceiveByte();
I2C_Nack();
I2C_Stop();
value = (int16_t)((data[1] << 8) | data[0]);
return value;
}
void bme280_init(void)
{
// Set BME280 to normal mode with 16x oversampling for temperature,
// 16x oversampling for humidity, and 16x oversampling for pressure
bme280_write_byte(0xF2, 0x01);
bme280_write_byte(0xF4, 0x27);
}
void bme280_read_data(int32_t *temperature, uint32_t *humidity, uint32_t *pressure)
{
int32_t var1, var2, t_fine;
int32_t adc_T, adc_H, adc_P;
adc_T = bme280_read_short(0xFA);
adc_H = (uint32_t)bme280_read_short(0xFD);
adc_P = (uint32_t)bme280_read_short(0xF7);
var1 = ((((adc_T >> 3) - ((int32_t)bme280_read_short(0x88) << 1))) *
((int32_t)bme280_read_short(0x8A))) >> 12;
var2 = ((((adc_T >> 4) - ((int32_t)bme280_read_short(0x88))) *
((adc_T >> 4) - ((int32_t)bme280_read_short(0x88)))) >> 12) *
((int32_t)bme280_read_short(0x89)) >>
10;
t_fine = var1 + var2;
*temperature = (t_fine * 5 + 128) >> 8;
*humidity = (((adc_H >> 4) - ((int32_t)bme280_read_byte(0xA1) << 4) -
(((int32_t)bme280_read_byte(0xE1)) >> 4) + 16384) >>
15) *
(((((((t_fine)-76800) * (int32_t)bme280_read_byte(0xE3))) >> 7) +
((int32_t)bme280_read_byte(0xE4) << 16)) >>
2) +
1) >>
16;
*pressure = (((((adc_P >> 3) - ((int32_t)bme280_read_short(0x8E) << 1))) *
((int32_t)bme280_read_short(0x8F))) >>
12) +
(((((adc_P >> 2) - ((int32_t)bme280_read_short(0x8E))) *
((adc_P >> 2) - ((int32_t)bme280_read_short(0x8E)))) >>
13) *
((int32_t)bme280_read_short(0x90))) >>
16;
}
int main(void)
{
int32_t temperature;
uint32_t humidity, pressure;
I2C_Init();
bme280_init();
while (1)
{
bme280_read_data(&temperature, &humidity, &pressure);
// do something with the data
}
}
```
以上代码假设已经在STM32F103上实现了I2C接口的读写函数。在main函数中,首先调用bme280_init函数初始化BME280传感器,然后在循环中调用bme280_read_data函数读取温湿度气压数据,最后可以根据需要对数据进行处理。
相关推荐
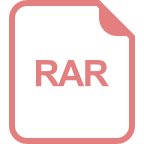
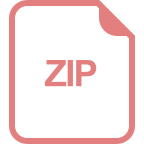














