zhuce::zhuce(QWidget *parent) : QWidget(parent), ui(new Ui::zhuce) { ui->setupUi(zhuce *); }
时间: 2024-04-04 16:34:36 浏览: 158
这是一个Qt的界面类 zhuce 的构造函数,它继承自 QWidget。其中,ui 是一个指向 Ui::zhuce 类对象的指针,它是通过 Qt 的用户界面设计器生成的。setupUi() 函数是用来初始化 zhuce 窗口的界面组件的,它会将所有的控件对象创建出来并设置其属性,并将它们放置在窗口上。在构造函数中,需要将当前的 zhuce 指针传递给 setupUi() 函数,这样才能正确地对界面进行初始化。
相关问题
给下面代码增加分页功能,要求细致,逻辑清楚,<form action="./../selectNews" method="post"> <input type="text" name="key" id="key" placeholder="请输入标题"/> <input type="submit" value="查询"/> <a href="zhuce.jsp">发布新闻</a> <a href="../user01/index.jsp">返回主页</a> </form> <table> <thead> <tr> <th>新闻编号</th> <th>新闻标题</th> <%-- <th>新闻内容</th>--%> <th>新闻类别</th> <th>发布人</th> <th>发布时间</th> <th>状态</th> <th>是否头条</th> <th colspan="4" align="center">操作</th> </tr> </thead> <tbody> <c:forEach items="${newslist}" var="u"> <tr> <td>${u.nid}</td> <td>${u.title}</td> <%-- <td>${u.content}</td>--%> <td>${u.type}</td> <td>${u.userName}</td> <td>${u.pubTime}</td> <td> <c:choose> <c:when test="${u.state==0}"><span style="color: ghostwhite">未审核</span></c:when> <c:when test="${u.state==1}"><span style="color: green">审核通过</span></c:when> <c:otherwise><span style="color: red">未通过</span></c:otherwise> </c:choose> </td> <td> <c:choose> <c:when test="${u.top==0}">否</c:when> <c:otherwise>是</c:otherwise> </c:choose> </td> <c:choose> <c:when test="${user.rule==1}"> <td> <a href="../NewsDetailServlet?nid=${u.nid}&op=show">查看</a> </td> <td> <a href="../NewsDetailServlet?nid=${u.nid}&op=update">修改</a> </td> <td> <a href="NewsDelete.jsp?nid=${u.nid}&title=${u.title}&userName=${user.userName}">删除</a> </td> <td> <a href="Newsshenhe.jsp?nid=${u.nid}&title=${u.title}&userName=${user.userName}">审核</a> </td> </c:when> <c:when test="${user.rule==0 && user.uid==u.uid}"> <td><a href="../NewsDetailServlet?nid=${u.nid}&op=show">查看</a></td> <td><a href="../NewsDetailServlet?nid=${u.nid}&op=update">修改</a></td> <td><a href="NewsDelete.jsp?nid=${u.nid}&title=${u.title}&userName=${user.userName}">删除</a></td> </c:when> <c:otherwise> <td><a href="../NewsDetailServlet?nid=${u.nid}&op=show">查看</a></td> </c:otherwise> </c:choose> </tr> </c:forEach> </table>
首先,我们需要在表单中添加一个分页控件,例如:
<form action="./../selectNews" method="post">
<input type="text" name="key" id="key" placeholder="请输入标题"/>
<input type="submit" value="查询"/>
<a href="zhuce.jsp">发布新闻</a>
<a href="../user01/index.jsp">返回主页</a>
<!-- 分页控件 -->
<div class="pagination">
<c:if test="${currentPage > 1}">
<a href="./../selectNews?page=${currentPage - 1}&key=${param.key}">上一页</a>
</c:if>
<c:forEach begin="${minPage}" end="${maxPage}" var="i">
<c:choose>
<c:when test="${i == currentPage}">
<span class="current">${i}</span>
</c:when>
<c:otherwise>
<a href="./../selectNews?page=${i}&key=${param.key}">${i}</a>
</c:otherwise>
</c:choose>
</c:forEach>
<c:if test="${currentPage < totalPages}">
<a href="./../selectNews?page=${currentPage + 1}&key=${param.key}">下一页</a>
</c:if>
</div>
</form>
我们使用了一个名为 "pagination" 的 div 元素来包含分页控件。其中,currentPage 表示当前页数,totalPages 表示总页数,minPage 和 maxPage 分别表示当前页数左边和右边的页数范围。
在 Java Servlet 中,我们需要对请求参数进行解析,计算出分页所需的各个参数,并将它们传递给 JSP 页面。例如:
int page = Integer.parseInt(request.getParameter("page"));
int pageSize = 10; // 每页显示的记录数
int start = (page - 1) * pageSize; // 计算起始记录数
String key = request.getParameter("key");
List<News> newslist = NewsDAO.selectNews(start, pageSize, key);
int totalCount = NewsDAO.getNewsCount(key);
int totalPages = (int) Math.ceil(totalCount * 1.0 / pageSize);
int minPage = Math.max(1, page - 5);
int maxPage = Math.min(totalPages, page + 5);
request.setAttribute("newslist", newslist);
request.setAttribute("currentPage", page);
request.setAttribute("totalPages", totalPages);
request.setAttribute("minPage", minPage);
request.setAttribute("maxPage", maxPage);
request.getRequestDispatcher("newslist.jsp").forward(request, response);
在 JSP 页面中,我们需要根据传递过来的参数来生成分页控件。我们可以使用 JSTL 标签库中的 forEach 标签来循环生成分页链接。例如:
<c:if test="${currentPage > 1}">
<a href="./../selectNews?page=${currentPage - 1}&key=${param.key}">上一页</a>
</c:if>
<c:forEach begin="${minPage}" end="${maxPage}" var="i">
<c:choose>
<c:when test="${i == currentPage}">
<span class="current">${i}</span>
</c:when>
<c:otherwise>
<a href="./../selectNews?page=${i}&key=${param.key}">${i}</a>
</c:otherwise>
</c:choose>
</c:forEach>
<c:if test="${currentPage < totalPages}">
<a href="./../selectNews?page=${currentPage + 1}&key=${param.key}">下一页</a>
</c:if>
注意,我们在分页链接中传递了当前页数和查询关键字两个参数,这样在用户点击分页链接时就可以保持查询关键字不变,同时跳转到相应的页数。
最后,我们需要在查询语句中添加 LIMIT 子句来限制返回的记录数。例如:
SELECT * FROM news WHERE title LIKE '%${key}%' LIMIT ${start}, ${pageSize};
其中,${start} 和 ${pageSize} 分别表示起始记录数和每页显示的记录数。这样就可以实现分页功能了。
<!DOCTYPE html> <html> <head> <title>注册页面</title> <link rel="stylesheet" type="text/css" href="../zhuce/zhuce1.css"> </head> <body> <h2>用户注册</h2> <form method="post" action='http://localhost:3000' id="registration-form"> <label for="username">用户名:</label> <input type="text" id="name" name="name" placeholder="请输入用户名" pattern="^[a-zA-Z0-9_]{3,20}$" required> <label for="email">邮箱:</label> <input type="email" id="email" name="email" placeholder="请输入邮箱地址" required> <label for="password">密码:</label> <input type="password" id="password" name="password" placeholder="请输入密码" pattern="^(?=.*\d)(?=.*[a-z])(?=.*[A-Z])(?=.*[a-zA-Z]).{8,}$" required> <input type="submit" value="注册"> </form> </body> </html> <script> document.addEventListener('DOMContentLoaded', function () { const form = document.getElementById('registration-form'); form.addEventListener('submit', function (event) { event.preventDefault(); // 阻止表单提交 const name = document.getElementById('name').value; const password = document.getElementById('password').value; const email = document.getElementById('email').value; console.log('注册信息:'); console.log('用户名:', name); console.log('密码:', password); console.log('邮箱:', email); }); }); </script>根据以上代码,写一个把注册页面的数据发送到sql sever的数据库
根据您提供的代码,以下是一个示例,展示如何将注册页面的数据发送到SQL Server数据库:
```html
<!DOCTYPE html>
<html>
<head>
<title>注册页面</title>
<link rel="stylesheet" type="text/css" href="../zhuce/zhuce1.css">
</head>
<body>
<h2>用户注册</h2>
<form method="post" action='http://localhost:3000/register' id="registration-form">
<label for="username">用户名:</label>
<input type="text" id="name" name="name" placeholder="请输入用户名" pattern="^[a-zA-Z0-9_]{3,20}$" required>
<label for="email">邮箱:</label>
<input type="email" id="email" name="email" placeholder="请输入邮箱地址" required>
<label for="password">密码:</label>
<input type="password" id="password" name="password" placeholder="请输入密码"
pattern="^(?=.*\d)(?=.*[a-z])(?=.*[A-Z])(?=.*[a-zA-Z]).{8,}$" required>
<input type="submit" value="注册">
</form>
<script>
document.addEventListener('DOMContentLoaded', function () {
const form = document.getElementById('registration-form');
form.addEventListener('submit', function (event) {
event.preventDefault(); // 阻止表单提交
const name = document.getElementById('name').value;
const password = document.getElementById('password').value;
const email = document.getElementById('email').value;
// 创建一个新的XMLHttpRequest对象
const xhr = new XMLHttpRequest();
// 设置POST请求的URL
xhr.open('POST', 'http://localhost:3000/register', true);
// 设置请求头
xhr.setRequestHeader('Content-type', 'application/x-www-form-urlencoded');
// 构建要发送的数据
const data = `name=${encodeURIComponent(name)}&email=${encodeURIComponent(email)}&password=${encodeURIComponent(password)}`;
// 处理请求完成时的回调
xhr.onload = function () {
if (xhr.status === 200) {
console.log('注册成功');
// 可以在这里执行其他处理,如显示成功消息或跳转到其他页面
} else {
console.log('注册失败');
// 可以在这里执行其他处理,如显示错误消息或进行其他错误处理
}
};
// 发送请求
xhr.send(data);
});
});
</script>
</body>
</html>
```
上述示例中,注册表单的提交按钮被点击时,JavaScript代码会阻止表单的默认提交行为,并使用XMLHttpRequest对象发送POST请求到指定的URL(这里假设URL为`http://localhost:3000/register`)。请求中包含了注册页面的用户名、邮箱和密码作为数据。
在服务器端,您需要使用适当的后端技术(例如Node.js中的Express框架)来接受该POST请求,并将接收到的数据插入到SQL Server数据库中。
请注意,上述代码只是一个示例,并且需要根据您的具体需求和技术栈进行适当修改和调整。同时,确保在处理用户输入时进行适当的验证和过滤,以提高安全性。
阅读全文
相关推荐
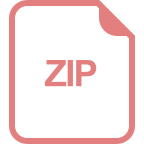
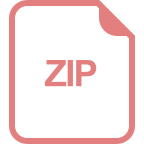











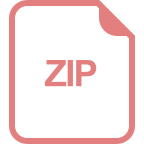
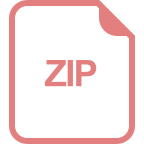