微信小程序通过蓝牙控制水阀开关代码示例
时间: 2023-08-20 13:05:02 浏览: 95
以下是一个简单的微信小程序蓝牙控制水阀开关的代码示例:
1. 在 app.json 中添加蓝牙权限声明:
```json
{
"permission": {
"bluetooth": {
"desc": "用于连接水阀设备"
}
}
}
```
2. 在页面中添加按钮,用于开关水阀:
```html
<button type="primary" bindtap="openValve">开阀</button>
<button type="primary" bindtap="closeValve">关阀</button>
```
3. 在页面的 js 文件中添加蓝牙连接与数据传输的代码:
```javascript
Page({
data: {
deviceId: '', // 设备 ID
serviceId: '', // 服务 ID
characteristicId: '', // 特征值 ID
connected: false // 连接状态
},
// 连接蓝牙设备
connectToDevice: function () {
wx.createBLEConnection({
deviceId: this.data.deviceId,
success: (res) => {
console.log('连接成功', res)
this.setData({
connected: true
})
// 获取服务列表
this.getServices()
},
fail: (err) => {
console.log('连接失败', err)
}
})
},
// 获取服务列表
getServices: function () {
wx.getBLEDeviceServices({
deviceId: this.data.deviceId,
success: (res) => {
console.log('获取服务列表成功', res)
// 遍历服务列表,找到需要的服务
res.services.forEach(service => {
if (service.uuid === this.data.serviceId) {
// 获取特征值列表
this.getCharacteristics(service.uuid)
}
})
},
fail: (err) => {
console.log('获取服务列表失败', err)
}
})
},
// 获取特征值列表
getCharacteristics: function (serviceId) {
wx.getBLEDeviceCharacteristics({
deviceId: this.data.deviceId,
serviceId: serviceId,
success: (res) => {
console.log('获取特征值列表成功', res)
// 遍历特征值列表,找到需要的特征值
res.characteristics.forEach(characteristic => {
if (characteristic.uuid === this.data.characteristicId) {
// 监听特征值变化
this.notifyCharacteristicValueChange(characteristic.uuid)
}
})
},
fail: (err) => {
console.log('获取特征值列表失败', err)
}
})
},
// 监听特征值变化
notifyCharacteristicValueChange: function (characteristicId) {
wx.notifyBLECharacteristicValueChange({
deviceId: this.data.deviceId,
serviceId: this.data.serviceId,
characteristicId: characteristicId,
state: true,
success: (res) => {
console.log('监听特征值变化成功', res)
},
fail: (err) => {
console.log('监听特征值变化失败', err)
}
})
},
// 发送数据
sendData: function (data) {
wx.writeBLECharacteristicValue({
deviceId: this.data.deviceId,
serviceId: this.data.serviceId,
characteristicId: this.data.characteristicId,
value: data,
success: (res) => {
console.log('发送数据成功', res)
},
fail: (err) => {
console.log('发送数据失败', err)
}
})
},
// 断开蓝牙连接
disconnect: function () {
wx.closeBLEConnection({
deviceId: this.data.deviceId,
success: (res) => {
console.log('断开连接成功', res)
this.setData({
connected: false
})
},
fail: (err) => {
console.log('断开连接失败', err)
}
})
},
// 开阀
openValve: function () {
if (this.data.connected) {
// 发送开阀指令
this.sendData('open')
} else {
// 连接蓝牙设备
this.connectToDevice()
}
},
// 关阀
closeValve: function () {
if (this.data.connected) {
// 发送关阀指令
this.sendData('close')
} else {
// 连接蓝牙设备
this.connectToDevice()
}
}
})
```
注意:上述代码中的 deviceId、serviceId 和 characteristicId 需要替换为实际的值。另外,开阀和关阀指令的格式和内容需要与水阀设备的协议一致。
相关推荐
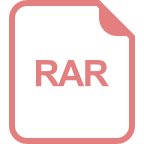














