写一个基于uc操作系统的OLED 代码
时间: 2024-04-08 11:34:14 浏览: 11
Sure! Here is a sample code for driving an OLED display using the UCOS-II operating system:
```c
#include "ucos_ii.h"
#include "oled.h"
#define TASK_OLED_PRIO 3
#define TASK_OLED_STACK_SIZE 128
OS_STK TaskOLEDStack[TASK_OLED_STACK_SIZE];
void TaskOLED(void* pdata);
int main(void)
{
OSInit(); // Initialize UCOS-II
// Create the OLED task
OSTaskCreate(TaskOLED, NULL, &TaskOLEDStack[TASK_OLED_STACK_SIZE - 1], TASK_OLED_PRIO);
OSStart(); // Start UCOS-II
return 0;
}
void TaskOLED(void* pdata)
{
(void)pdata; // Avoid compiler warning about unused parameter
// Initialize OLED display
OLED_Init();
while (1)
{
// Update OLED display content here
OSTimeDlyHMSM(0, 0, 1, 0); // Delay for 1 second
}
}
```
Please note that this code assumes you have already implemented the necessary driver functions for the OLED display (e.g., `OLED_Init()`). The code creates a task (`TaskOLED`) that is responsible for updating the content on the OLED display. The task runs in an infinite loop, updating the display and then delaying for 1 second using `OSTimeDlyHMSM()` function.
Remember to include the necessary header files and adapt the code to your specific hardware setup and requirements.
相关推荐
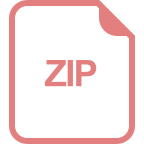
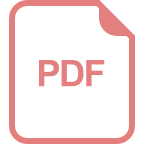
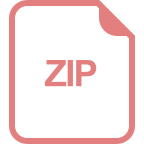














