生成基于VGG16回归模型的Grad-CAM PyTorch实现代码
时间: 2024-01-24 12:18:38 浏览: 155
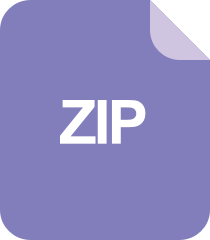
Grad-CAM的Pytorch实现-python
以下是基于 VGG16 回归模型的 Grad-CAM PyTorch 实现代码示例:
```python
import torch
import torchvision.models as models
import torch.nn.functional as F
import cv2
import numpy as np
# Load pre-trained VGG16 model
model = models.vgg16(pretrained=True)
# Define the last convolutional layer of VGG16
target_layer = model.features[-1]
# Define the input tensor
input_tensor = torch.randn(1, 3, 224, 224, requires_grad=True)
# Define the class index for which the Grad-CAM will be computed
class_index = 10
# Forward pass through the model
output = model(input_tensor)
# Compute the gradients of the output with respect to the input tensor
output[:, class_index].backward()
# Get the gradients of the target layer
gradients = target_layer.weight.grad
# Compute the average gradients across all spatial dimensions
pooled_gradients = torch.mean(gradients, dim=[2, 3])
# Get the activations of the target layer
activations = model.features(input_tensor)
# Compute the weighted sum of the activations and gradients
for i in range(512):
activations[:, i, :, :] *= pooled_gradients[0, i]
# Get the heatmap
heatmap = torch.mean(activations, dim=1).squeeze().detach().numpy()
# Apply ReLU to the heatmap
heatmap = np.maximum(heatmap, 0)
# Normalize the heatmap
heatmap /= np.max(heatmap)
# Load the original image
img = cv2.imread('path/to/image.jpg')
# Resize the heatmap to the size of the original image
heatmap = cv2.resize(heatmap, (img.shape[1], img.shape[0]))
# Convert the heatmap to RGB
heatmap = np.uint8(255 * heatmap)
heatmap = cv2.applyColorMap(heatmap, cv2.COLORMAP_JET)
# Overlay the heatmap on the original image
result = cv2.addWeighted(img, 0.8, heatmap, 0.4, 0)
# Display the result
cv2.imshow('Grad-CAM', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
注意:以上代码仅供参考,具体实现可能需要根据自己的数据和模型做一些调整。
阅读全文
相关推荐
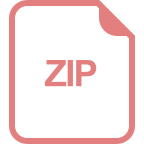

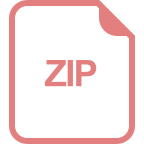

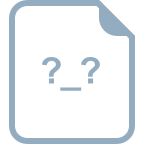
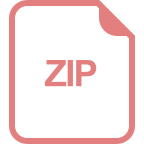
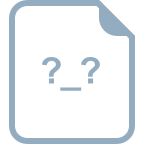
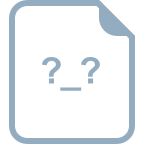
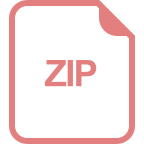
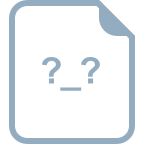
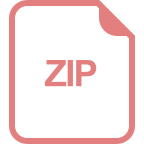
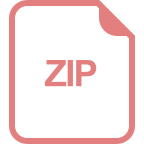
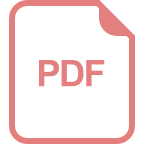
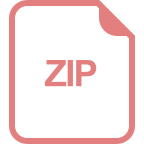
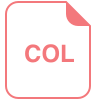
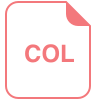
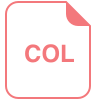