switch case 字符串
时间: 2023-07-24 07:38:24 浏览: 48
在 C++ 11 之后,支持使用字符串作为 switch case 条件。具体实现方式可以使用 std::string 或者 char[] 类型来表示字符串,然后使用常量表达式来作为 case 条件。下面是一个示例代码:
```
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "hello";
switch (str[0]) {
case 'h':
cout << "The first letter is h" << endl;
break;
case 'w':
cout << "The first letter is w" << endl;
break;
default:
cout << "The first letter is neither h nor w" << endl;
}
return 0;
}
```
在上面的代码中,我们使用 std::string 类型表示字符串,并且使用 str[0] 来获取字符串的第一个字符。然后我们使用 switch case 来判断第一个字符是不是 'h' 或者 'w'。如果是,就输出对应的信息,否则输出默认信息。
相关问题
switch case字符串
在 C++ 中,`switch case` 语句也可以用于比较字符串。然而,C++ 中的 `switch case` 语句只支持整数类型的表达式,所以直接使用字符串作为表达式是不被允许的。
为了在 `switch case` 语句中比较字符串,你可以使用 `strcmp()` 函数来比较字符串的值。下面是一个示例:
```C++
#include <iostream>
#include <cstring>
int main() {
char str[] = "apple";
if (strcmp(str, "apple") == 0) {
std::cout << "It's an apple!" << std::endl;
} else if (strcmp(str, "banana") == 0) {
std::cout << "It's a banana!" << std::endl;
} else {
std::cout << "It's neither an apple nor a banana." << std::endl;
}
return 0;
}
```
在上面的示例中,我们使用 `strcmp()` 函数来比较字符串 `str` 和 `"apple"`、`"banana"` 的值。如果两个字符串相等,则返回值为 0,因此我们可以使用 `strcmp() == 0` 来进行比较。请注意,在使用 `strcmp()` 比较字符串时,需要包含头文件 `<cstring>`。
switchcase 判断字符串
在JavaScript中,switch case语句可以用于判断字符串。但是需要注意的是,switch case语句在比较时使用的是全等号“===”,因此需要确保每个case后面的字符串与待比较的字符串类型和值都完全相同。下面是一个例子:
```
switch(str) {
case "apple":
// do something
break;
case "banana":
// do something
break;
default:
// do something
}
```
在这个例子中,如果str的值为"apple"或"banana",则会执行相应的代码块。如果str的值不是这两个字符串中的任何一个,则会执行default代码块。需要注意的是,每个case后面的代码块必须以break语句结束,否则会继续执行下一个case的代码块。
相关推荐
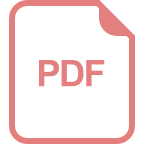
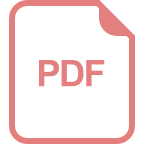
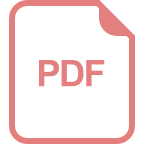












