EDK2编写UEFI代码,判断文件中是否存在某字符串
时间: 2024-05-13 17:21:02 浏览: 157
要判断文件中是否存在某字符串,可以使用UEFI提供的文件访问协议和Unicode字符串处理函数。
1. 打开文件访问协议
使用EFI_SIMPLE_FILE_SYSTEM_PROTOCOL打开文件访问协议,获取EFI_FILE_PROTOCOL指针。
2. 定位文件位置
使用EFI_FILE_PROTOCOL的SetPosition()函数定位文件读取位置。
3. 读取文件内容
使用EFI_FILE_PROTOCOL的Read()函数读取文件内容。
4. 判断字符串是否存在
使用Unicode字符串处理函数StrStr(),判断读取出的文件内容中是否包含目标字符串。
以下是一份示例代码:
```c
#include <Uefi.h>
#include <Library/UefiLib.h>
#include <Library/UefiBootServicesTableLib.h>
#include <Protocol/SimpleFileSystem.h>
EFI_STATUS
EFIAPI
UefiMain(IN EFI_HANDLE ImageHandle, IN EFI_SYSTEM_TABLE *SystemTable)
{
EFI_STATUS Status;
// 获取文件系统协议
EFI_SIMPLE_FILE_SYSTEM_PROTOCOL *SimpleFileSystem;
Status = gBS->LocateProtocol(&gEfiSimpleFileSystemProtocolGuid, NULL, (VOID **)&SimpleFileSystem);
if (EFI_ERROR(Status)) {
Print(L"Failed to locate file system protocol. (status = %r)\n", Status);
return Status;
}
// 打开文件
EFI_FILE_PROTOCOL *File;
Status = SimpleFileSystem->OpenVolume(SimpleFileSystem, &File);
if (EFI_ERROR(Status)) {
Print(L"Failed to open volume. (status = %r)\n", Status);
return Status;
}
// 定位文件位置
Status = File->SetPosition(File, 0);
if (EFI_ERROR(Status)) {
Print(L"Failed to set file position. (status = %r)\n", Status);
return Status;
}
// 读取文件内容
UINTN BufferSize = 1024;
CHAR8 Buffer[BufferSize];
Status = File->Read(File, &BufferSize, Buffer);
if (EFI_ERROR(Status)) {
Print(L"Failed to read file. (status = %r)\n", Status);
return Status;
}
// 判断字符串是否存在
CHAR8 *TargetString = "Hello, world!";
CHAR8 *Result = NULL;
Result = (CHAR8 *)StrStr((CHAR16 *)Buffer, (CHAR16 *)TargetString);
if (Result != NULL) {
Print(L"The string '%a' was found in the file.\n", TargetString);
} else {
Print(L"The string '%a' was not found in the file.\n", TargetString);
}
// 关闭文件
Status = File->Close(File);
if (EFI_ERROR(Status)) {
Print(L"Failed to close file. (status = %r)\n", Status);
return Status;
}
return EFI_SUCCESS;
}
```
阅读全文
相关推荐
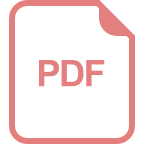
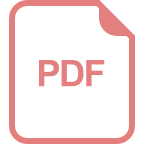
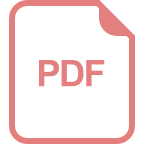















