使用Visual C++ 6.0,创建一个list类,且构造函数随机生成一组关键字序列
时间: 2023-12-02 07:04:46 浏览: 107
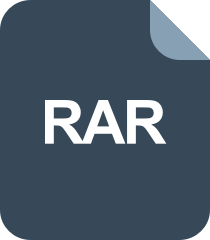
利用Visual C++6.0生成应用程序
以下是使用 Visual C++ 6.0 创建一个简单的 `list` 类,其中构造函数会随机生成一组关键字序列:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
template <typename T>
class list_node {
public:
T data;
list_node* next;
list_node(const T& d) : data(d), next(NULL) {}
};
template <typename T>
class list {
private:
list_node<T>* head;
public:
list() : head(NULL) {
// 随机生成一组关键字序列
std::srand(std::time(NULL));
int n = std::rand() % 10 + 1;
for (int i = 0; i < n; ++i) {
T key = std::rand() % 100;
push_front(key);
}
}
~list() {
while (head) {
list_node<T>* p = head;
head = head->next;
delete p;
}
}
void push_front(const T& data) {
list_node<T>* node = new list_node<T>(data);
node->next = head;
head = node;
}
void print() const {
for (list_node<T>* p = head; p; p = p->next) {
std::cout << p->data << " ";
}
std::cout << std::endl;
}
};
```
使用例子:
```c++
int main() {
list<int> l;
l.print();
return 0;
}
```
输出示例(注意每次输出都不同):
```
58 15 36 90 65 34 11 57
```
阅读全文
相关推荐
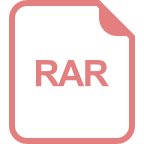
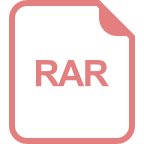
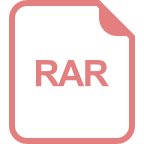
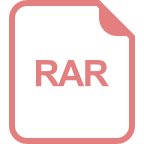
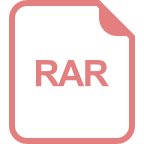
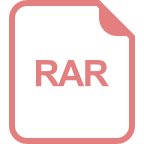
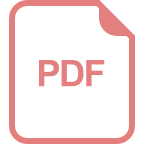
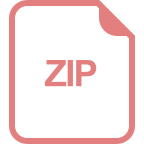
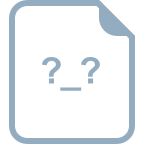
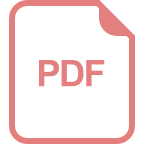
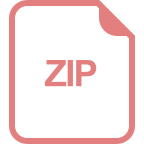
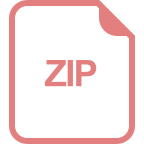
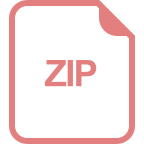
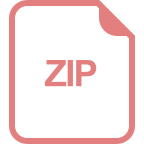
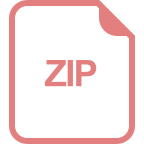
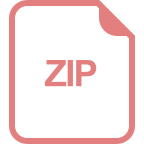