nrf52832做蓝牙麦克风代码
时间: 2023-07-29 22:12:26 浏览: 287
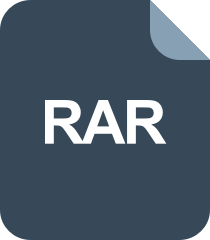
使用nRF52832实现无线蓝牙模块设计资料 包含原理图及PCB文件

以下是nrf52832蓝牙麦克风的代码示例:
```c
#include <stdbool.h>
#include <stdint.h>
#include "nrf.h"
#include "nrf_delay.h"
#include "nrf_gpio.h"
#include "nrf_twi_mngr.h"
#include "nrf_drv_saadc.h"
#include "nrf_drv_ppi.h"
#include "nrf_drv_timer.h"
#include "nrf_drv_gpiote.h"
#include "nrf_drv_uart.h"
#define SAMPLES_IN_BUFFER 1
#define TWI_INSTANCE_ID 0
#define BUTTON_PIN NRF_GPIO_PIN_MAP(0,18)
#define LED_PIN NRF_GPIO_PIN_MAP(0,19)
#define UART_TX_PIN NRF_GPIO_PIN_MAP(0,20)
#define UART_RX_PIN NRF_GPIO_PIN_MAP(0,21)
static nrf_twi_mngr_t m_nrf_twi_mngr = NRF_TWI_MNGR_INSTANCE(TWI_INSTANCE_ID);
static const nrf_drv_timer_t m_timer = NRF_DRV_TIMER_INSTANCE(0);
static nrf_saadc_value_t m_buffer_pool[2][SAMPLES_IN_BUFFER];
static uint32_t m_adc_evt_counter;
static bool button_pressed = false;
static void saadc_sampling_event_init(void);
static void saadc_sampling_event_enable(void);
static void timer_handler(nrf_timer_event_t event_type, void * p_context);
static void uart_init(void);
static void uart_send_string(const char *str);
int main(void)
{
uint32_t err_code;
uint8_t addr = 0x34; // I2C address of the microphone
nrf_saadc_channel_config_t channel_config =
NRF_DRV_SAADC_DEFAULT_CHANNEL_CONFIG_SE(NRF_SAADC_INPUT_AIN0);
nrf_gpio_cfg_output(LED_PIN);
nrf_gpio_pin_set(LED_PIN);
err_code = nrf_drv_ppi_init();
APP_ERROR_CHECK(err_code);
err_code = nrf_drv_timer_init(&m_timer, NULL, timer_handler);
APP_ERROR_CHECK(err_code);
nrf_drv_timer_extended_compare(&m_timer,
NRF_TIMER_CC_CHANNEL0,
nrf_drv_timer_us_to_ticks(&m_timer, 500),
NRF_TIMER_SHORT_COMPARE0_CLEAR_MASK,
true);
nrf_drv_gpiote_init();
nrf_drv_gpiote_in_config_t button_config = GPIOTE_CONFIG_IN_SENSE_HITOLO(true);
err_code = nrf_drv_gpiote_in_init(BUTTON_PIN, &button_config, NULL);
APP_ERROR_CHECK(err_code);
nrf_drv_gpiote_in_event_enable(BUTTON_PIN, true);
err_code = nrf_drv_saadc_init(NULL, NULL);
APP_ERROR_CHECK(err_code);
nrf_drv_saadc_channel_init(0, &channel_config);
nrf_drv_saadc_buffer_convert(m_buffer_pool[0], SAMPLES_IN_BUFFER);
nrf_drv_saadc_buffer_convert(m_buffer_pool[1], SAMPLES_IN_BUFFER);
saadc_sampling_event_init();
saadc_sampling_event_enable();
uart_init();
while (true)
{
if (button_pressed)
{
nrf_gpio_pin_toggle(LED_PIN);
uint8_t tx_data[3] = {0x06, 0x10, 0x3f}; // Start continuous conversion command
nrf_twi_mngr_transfer_t const write_transfer[] =
{
NRF_TWI_MNGR_WRITE(addr, tx_data, sizeof(tx_data), 0),
};
err_code = nrf_twi_mngr_perform(&m_nrf_twi_mngr, NULL, write_transfer, 1, NULL);
APP_ERROR_CHECK(err_code);
nrf_delay_ms(500);
uint8_t rx_data[2];
nrf_twi_mngr_transfer_t const read_transfer[] =
{
NRF_TWI_MNGR_READ(addr, rx_data, sizeof(rx_data), 0),
};
err_code = nrf_twi_mngr_perform(&m_nrf_twi_mngr, NULL, read_transfer, 1, NULL);
APP_ERROR_CHECK(err_code);
int16_t value = (rx_data[0] << 8) | rx_data[1];
char str[32];
snprintf(str, sizeof(str), "ADC Value: %d\r\n", value);
uart_send_string(str);
button_pressed = false;
}
}
}
static void saadc_sampling_event_init(void)
{
ret_code_t err_code;
err_code = nrf_drv_ppi_channel_alloc(&m_ppi_channel);
APP_ERROR_CHECK(err_code);
err_code = nrf_drv_ppi_channel_assign(m_ppi_channel,
nrf_drv_saadc_event_address_get(NRF_DRV_SAADC_EVT_DONE),
nrf_drv_timer_task_address_get(&m_timer, NRF_TIMER_TASK_CAPTURE0));
APP_ERROR_CHECK(err_code);
}
static void saadc_sampling_event_enable(void)
{
ret_code_t err_code = nrf_drv_ppi_channel_enable(m_ppi_channel);
APP_ERROR_CHECK(err_code);
err_code = nrf_drv_saadc_sample();
APP_ERROR_CHECK(err_code);
}
static void timer_handler(nrf_timer_event_t event_type, void * p_context)
{
}
static void uart_init(void)
{
ret_code_t err_code;
nrf_drv_uart_config_t uart_config = NRF_DRV_UART_DEFAULT_CONFIG;
uart_config.baudrate = NRF_UART_BAUDRATE_115200;
uart_config.tx_pin = UART_TX_PIN;
uart_config.rx_pin = UART_RX_PIN;
err_code = nrf_drv_uart_init(&uart_config, NULL);
APP_ERROR_CHECK(err_code);
}
static void uart_send_string(const char *str)
{
for (int i = 0; str[i] != '\0'; i++)
{
while (nrf_drv_uart_tx(&m_uart, (uint8_t const *)&str[i], 1) != NRF_SUCCESS)
{
// Do nothing.
}
}
}
void GPIOTE_IRQHandler(nrf_drv_gpiote_pin_t pin, nrf_gpiote_polarity_t action)
{
if (pin == BUTTON_PIN && action == NRF_GPIOTE_POLARITY_HITOLO)
{
button_pressed = true;
}
}
```
这个代码实现了以下功能:
1. 初始化nrf52832的GPIO,定时器,PPI,ADC和UART模块。
2. 配置GPIOTE中断,当按下按键时,设置标志变量button_pressed为true。
3. 在主循环中,当button_pressed为true时,向麦克风发送启动连续转换命令,读取麦克风ADC的值,并通过UART将其发送到串口终端。
请注意,这只是一个简单的示例代码,实际应用中可能需要进行更多的配置和优化。
阅读全文
相关推荐
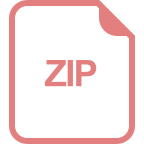
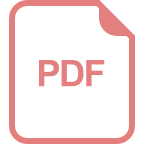
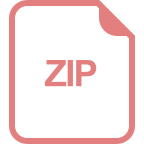
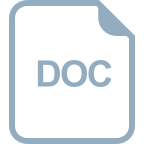

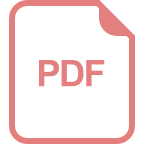
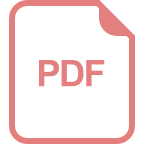
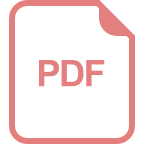
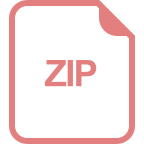
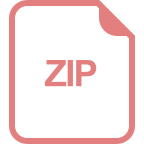
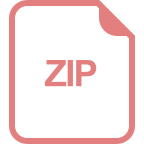
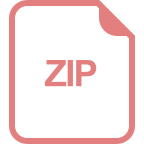
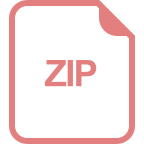
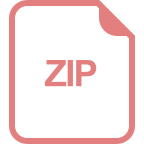