c++和linux指定一个路径,遍历路径下所有文件和目录,并输出文件和目录的名称
时间: 2024-03-03 11:47:23 浏览: 46
可以使用C++的文件流和Linux的文件系统API来实现这个功能。以下是一个示例代码:
```c++
#include <iostream>
#include <fstream>
#include <dirent.h>
#include <sys/stat.h>
using namespace std;
void traverseDir(string path){
DIR *dir = opendir(path.c_str()); // 打开目录
if(!dir){
cerr << "Failed to open directory " << path << endl;
return;
}
struct dirent *entry;
while((entry = readdir(dir)) != NULL){ // 遍历目录
string name = entry->d_name;
if(name == "." || name == "..") continue;
string fullpath = path + "/" + name;
struct stat st;
if(stat(fullpath.c_str(), &st) == -1) continue;
if(S_ISDIR(st.st_mode)){ // 目录
cout << "[dir] " << name << endl;
traverseDir(fullpath); // 递归遍历子目录
} else if(S_ISREG(st.st_mode)){ // 文件
cout << "[file] " << name << endl;
}
}
closedir(dir);
}
int main(){
string path = "/path/to/directory";
traverseDir(path);
return 0;
}
```
这个程序会遍历指定路径下的所有文件和目录,并输出它们的名称。如果是目录,会递归遍历子目录。
相关推荐
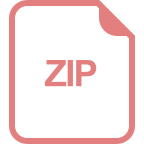
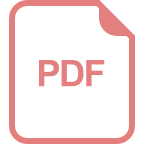














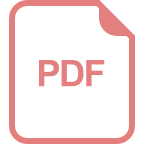