c++实现
时间: 2023-09-13 07:12:32 浏览: 51
以下是一个简单的 C++ 代码示例,可以实现复制文件和利用磁盘文件管理学生情况:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <map>
using namespace std;
// 复制文件
void CopyFile(const string& src_file, const string& dst_dir)
{
string dst_file = dst_dir + "/" + src_file.substr(src_file.find_last_of('/') + 1);
ifstream src(src_file, ios::binary);
ofstream dst(dst_file, ios::binary);
dst << src.rdbuf();
}
// 利用磁盘文件管理学生情况
void ManageStudents()
{
map<string, map<string, string>> students = {
{"student1", {{"name", "Alice"}, {"age", "20"}, {"gender", "female"}}},
{"student2", {{"name", "Bob"}, {"age", "21"}, {"gender", "male"}}},
};
// 写入学生信息到文件
ofstream outfile("students.txt");
for (auto& student : students) {
outfile << student.first << ": ";
for (auto& prop : student.second) {
outfile << prop.first << "=" << prop.second << " ";
}
outfile << endl;
}
// 从文件读取学生信息
ifstream infile("students.txt");
string line;
while (getline(infile, line)) {
string student_id = line.substr(0, line.find(':'));
string props = line.substr(line.find(':') + 2);
size_t pos = 0;
map<string, string> student_props;
while ((pos = props.find(' ')) != string::npos) {
string prop = props.substr(0, pos);
string prop_name = prop.substr(0, prop.find('='));
string prop_value = prop.substr(prop.find('=') + 1);
student_props[prop_name] = prop_value;
props.erase(0, pos + 1);
}
students[student_id] = student_props;
}
for (auto& student : students) {
cout << student.first << ": ";
for (auto& prop : student.second) {
cout << prop.first << "=" << prop.second << " ";
}
cout << endl;
}
}
int main()
{
// 复制文件
string src_file = "path/to/source/file";
string dst_dir = "path/to/destination/directory";
CopyFile(src_file, dst_dir);
// 利用磁盘文件管理学生情况
ManageStudents();
return 0;
}
```
请注意,此代码示例仅用于演示目的,实际使用时需要根据具体需求进行适当修改。另外,为了保护学生隐私,实际使用时应该使用合适的加密和访问控制措施来保护敏感信息。
相关推荐
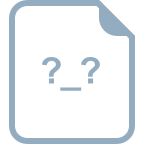
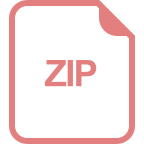















