前端手写轮播图
时间: 2023-06-28 07:15:44 浏览: 115
好的,以下是一个简单的前端手写轮播图实现思路:
1. HTML 结构中需要一个容器元素,用来包含轮播图。
2. 在该容器元素中,添加多个轮播项,每个轮播项包含一张图片和一段描述文字。
3. 通过 CSS 设置轮播项的样式,包括宽度、高度、位置等。
4. 在 JavaScript 中获取容器元素和轮播项元素,以及左右切换按钮元素。
5. 通过设置 CSS 样式,将当前显示的轮播项置于最前面。
6. 给左右切换按钮元素添加事件监听器,当点击时,通过 JavaScript 移动轮播项的位置,完成切换。
7. 使用定时器 setInterval() 实现自动轮播,每隔一定时间切换到下一个轮播项。
以下是一个简单的前端手写轮播图代码示例:
```html
<div class="carousel-container">
<div class="carousel-item active">
<img src="image1.jpg" alt="image1">
<div class="carousel-caption">
<h3>Image 1</h3>
<p>Description 1</p>
</div>
</div>
<div class="carousel-item">
<img src="image2.jpg" alt="image2">
<div class="carousel-caption">
<h3>Image 2</h3>
<p>Description 2</p>
</div>
</div>
<div class="carousel-item">
<img src="image3.jpg" alt="image3">
<div class="carousel-caption">
<h3>Image 3</h3>
<p>Description 3</p>
</div>
</div>
<div class="carousel-item">
<img src="image4.jpg" alt="image4">
<div class="carousel-caption">
<h3>Image 4</h3>
<p>Description 4</p>
</div>
</div>
<div class="carousel-item">
<img src="image5.jpg" alt="image5">
<div class="carousel-caption">
<h3>Image 5</h3>
<p>Description 5</p>
</div>
</div>
<div class="carousel-prev"></div>
<div class="carousel-next"></div>
</div>
```
```css
.carousel-container {
position: relative;
width: 800px;
height: 500px;
overflow: hidden;
}
.carousel-item {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
opacity: 0;
transition: all 1s ease-in-out;
}
.carousel-item.active {
opacity: 1;
}
.carousel-item img {
width: 100%;
height: 100%;
object-fit: cover;
}
.carousel-caption {
position: absolute;
bottom: 0;
left: 0;
width: 100%;
padding: 20px;
background-color: rgba(0, 0, 0, 0.5);
color: #fff;
font-size: 24px;
line-height: 1.5;
}
.carousel-prev,
.carousel-next {
position: absolute;
top: 50%;
transform: translateY(-50%);
width: 50px;
height: 50px;
background-color: rgba(0, 0, 0, 0.5);
cursor: pointer;
}
.carousel-prev {
left: 0;
}
.carousel-next {
right: 0;
}
```
```javascript
const carouselContainer = document.querySelector('.carousel-container');
const carouselItems = document.querySelectorAll('.carousel-item');
const prevBtn = document.querySelector('.carousel-prev');
const nextBtn = document.querySelector('.carousel-next');
let currentIndex = 0;
function showItem(index) {
carouselItems.forEach(item => {
item.classList.remove('active');
});
carouselItems[index].classList.add('active');
}
prevBtn.addEventListener('click', () => {
currentIndex--;
if (currentIndex < 0) {
currentIndex = carouselItems.length - 1;
}
showItem(currentIndex);
});
nextBtn.addEventListener('click', () => {
currentIndex++;
if (currentIndex > carouselItems.length - 1) {
currentIndex = 0;
}
showItem(currentIndex);
});
setInterval(() => {
currentIndex++;
if (currentIndex > carouselItems.length - 1) {
currentIndex = 0;
}
showItem(currentIndex);
}, 5000);
```
这是一个简单的轮播图实现,具体的实现方式可以根据实际需求进行调整。
阅读全文
相关推荐


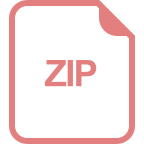
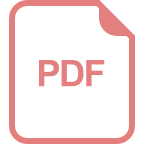
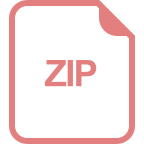
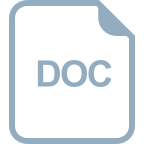
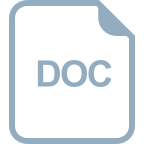
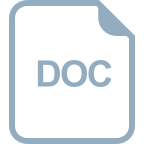
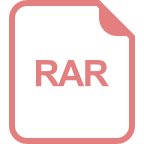
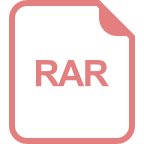
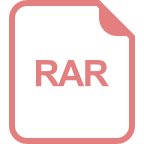
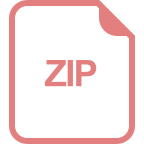
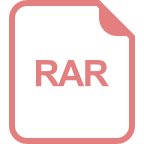
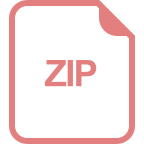